Open API V2 Document
Please note: This document is for Open API V2. For V1, please visit this link.
1. Start to Use
1.1 Preparation
Step 1. Please make sure the gateway is powered on and works properly.
The BLUE indicator is a normal network connection.
The RED indicator is an abnormal network connection.
Step 2. Make sure the gateway and the PC are connected to the same LAN. Then enter the URL http://ihost.local on your browser.
Notice:
If you have several gateways, you can get the corresponding IP address to access the specified gateway in the below two ways.
1. Log in to your wireless router and check the IP address of the gateway in DHCP.
2. iHost supports local discovery via mDNS.
1.2 Get started
- Call the [Access Token] interface, and get an error response, prompting to click <Done>.
Note that after pressing, the interface access is valid for no more than 5 minutes.
// Request
curl --location --request GET 'http://<ip address>/open-api/v2/rest/bridge/access_token' --header 'Content-Type: application/json'
// Response
{
"error": 401,
"data": {},
"message": "link button not pressed"
}
- Click <Done> and call the [Access Token] interface again. The response is successful, and the token is obtained.
// Request
curl --location --request GET 'http://<ip address>/open-api/v2/rest/bridge/access_token' --header 'Content-Type: application/json'
// Response
{
"error": 0,
"data": {
"token": "376310da-7adf-4521-b18c-5e0752cfff8d"
},
"message": "success"
}
- Verify token. Call the [Get Device List] interface. The response is successful, and the device list is obtained.
// Request
curl --location --request GET 'http://<ip address>/open-api/v2/rest/devices' \
--header 'Content-Type: application/json' \
--header 'Authorization: Bearer 376310da-7adf-4521-b18c-5e0752cfff8d'
// Response
{
"error": 0,
"data": {
"device_list": [
{
"serial_number": "ABCDEFGHIJK",
"name": "device name",
"manufacturer": "manufacturer name",
"model": "model name",
"firmware_version": "1.1.0",
"display_category": "switch",
"capabilities": [
{
"capability": "power",
"permission": "readWrite"
}
],
"protocal": "zigbee",
"state": {
"power": {
"powerState": "on"
}
},
"tags": {
"key": "value"
},
"online": true
}
]
}
"message": "success"
}
- Get iHost access token method: After calling the [Access Token] interface, the iHost Web console page global pop-up box prompts the user to confirm the acquisition of the interface call credentials.
- Note: If you open more than one iHost web console page, the confirmation pop-up box will appear on multiple web console pages together, and the pop-up box of other web console pages will be closed after clicking the confirmation on one of the web console pages.
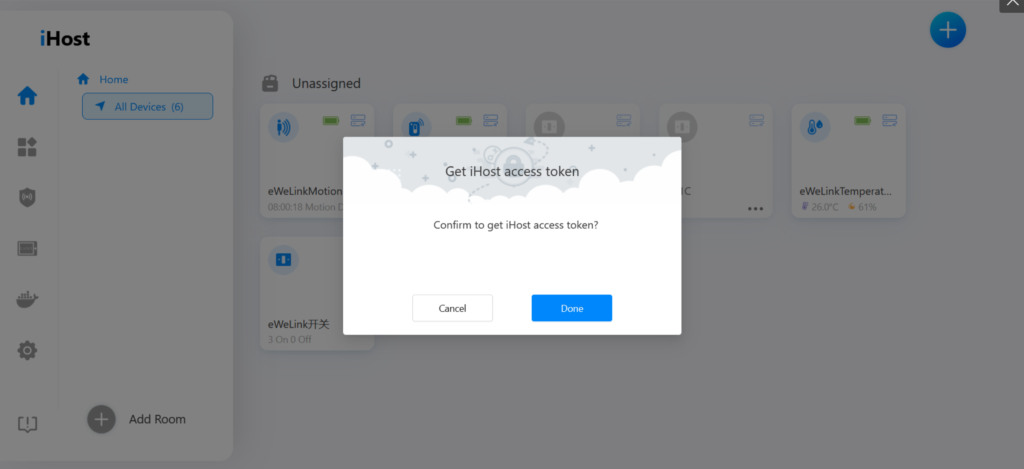
2. Core Concept
2.1 Development Interface Address
The gateway Web API interface has two access methods (based on IP or domain name), usually the root path is /open-api/v2/rest/< specific function module >
// IP access
http://<IP address>/open-api/v2/rest/bridge
// Domain name access
http://<domain address>/open-api/v2/rest/bridge
2.2 Device Display Category & Capabilities
- Device display category (DisplayCategory). Device display category is used to identify (device) specific categories, such as switch, plug, light, etc. This information will determine the UI display effect of the device in the gateway.
- Device Capability. Device capability is used to describe the specific sub-functions of the device. Such as power control, brightness control, color temperature control, etc. A single device can support 1 or more capabilities.
-
- capability: Describes the capability name, which must be globally unique and of string type. Multiple English words should be separated by hyphens (“-“). For example:
"thermostat-target-setpoint"
. - name: Describes the category under the capability, also of string type. Multiple English words should be separated by hyphens (“-“). For example:
"auto-mode"
,"manual-mode"
. - permission: Describes the permissions associated with the capability. The type is string, formatted in a 8421 binary code. Examples include:
- capability: Describes the capability name, which must be globally unique and of string type. Multiple English words should be separated by hyphens (“-“). For example:
-
- Device controllable:
"1000"
- Device configurable:
"0010"
- Device controllable and configurable:
"1010"
- Device controllable and reportable:
"1100"
- Device controllable:
The significance of each bit, from right to left, is as follows:
ⅰ. Bit 0: Allows querying the device
ⅱ. Bit 1: Allows configuring the device
ⅲ. Bit 2: Allows the device to report data
ⅳ. Bit 3: Allows controlling the device
const permission = {
"update": "1000",
"updated": "0100",
"configure": "0010",
"query": "0001",
"update-updated": "1100",
"update-query": "1001",
"update-updated-configure": "1110",
"updated-configure":"0110",
"update-updated-query":"1101"
}
settings:
Describes the configuration items for the capability, which are of object type and include a description of each configuration item.
ⅰ. key: Describes the name of the configuration item, which is of string type. Multiple English words should be expressed in camelCase. For example: "temperatureUnit"
.
ⅱ. value: Describes the content of the configuration item. The specific specifications are detailed in the table below.
Attribute |
Type |
Optional |
Description |
permission |
string |
N |
Describes the permissions for the configuration item. Optional values:
Bit explanation:
|
type |
string |
N |
Describes the data type of the configuration item value. Optional values:
Type-specific guidelines:
|
// type = enum
{
"settings":{
"temperatureUnit": {
"type": "enum",
"permission": "11",
"values": ["c", "f"],
"default": "c",
"value": "f"
}
}
}
// type = numeric
{
"settings": {
"setpointRange": {
"type": "numeric",
"permission": "01",
"min": 4,
"max": 35,
"default": 20,
"step": 0.5,
"precision": 0.01
"unit": "c"
}
}
}
// type = object
{
"settings":{
"weeklySchedule":{
"type": "object",
"permission": "11",
"value": {
"maxEntryPerDay": 2,
"Monday": [
{
"startTimeInMinutes": 440,
"upperSetpoint": 36.5
},
{
"startTimeInMinutes": 900,
"upperSetpoint": 26.5
}
],
"Tuesday": [...],
"Wednesday": [...],
"Thursday": [...],
"Friday": [...],
"Saturday": [...],
"Sunday": [...],
}
}
}
}
3.Web API
Type of Data
Type | Description |
string | String data type. UTF8 encoded. |
number | Number data type. double-precision 64-bit binary format IEEE 754 |
int | Integral data type. |
object | Object data type. JSON-compliant object |
string[] | Array of string |
int[] | Array of integral |
object[] | Array of object |
bool | Boolean |
date | Time string. String in ISO (ISO 8601 Extended Format) format: YYYY-MM-DDTHH:mm:ss.sssZ. The time zone is always UTC (Coordinated Universal Time), identified by a suffix “Z”. |
Generic Response Results
Attribute | Type | Optional | Description |
error | int | N | Error code: |
data | object | N | Response data body |
message | string | N | Response description: |
Response Example:
{
"error": 0,
"data": {
"token": "376310da-7adf-4521-b18c-5e0752cfff8d"
},
"message": "success"
}
{
"error": 400,
"data": {},
"message": "invalid parameters"
}
Resource List
Type | Description |
Supported sound | – alert1 (Alarm Sound 1) |
Supported deep | – bootComplete (System startup completed) |
3.1 The Gateway Function
a. Access Token
Allow users to access token.
- Notice: The token will be cleared after device reset.
- Notice: After obtaining the token, you need to press the button again to successfully obtain a new token.
- URL:
/open-api/v2/rest/bridge/access_token
- Method:
GET
- Header:
- Content-Type: application/json
Request Parameters:
Attribute | Type | Optional | Description |
app_name | string | N | Application name description. |
Successful data response:
Attribute | Type | Optional | Description |
token | string | N | The interface access token. It’s valid for a long time, please save it |
app_name | string | Y | Application name description. |
Condition: User trigger the < command key > and access this interface within the valid time.
Status Code: 200 OK
Response Example:
{
"error": 0,
"data": {
"token": "376310da-7adf-4521-b18c-5e0752cfff8d"
},
"message": "success"
}
Failure data response:empty Object
Conditions:The user has not triggered the < command key >, or the valid time has expired.
Status Code: 200 OK
Response Example:
{
"error": 401,
"data": {},
"message": "link button not pressed"
}
b. Get the State of Gateway
Allow authorized users to obtain the status of gateway through this interface
- URL:
/open-api/v2/rest/bridge/runtime
- Method:
GET
- Header:
- Content-Type: application/json
- Autorization: Bearer <token>
Request Parameters: none
Successful data response:
Attribute | Type | Optional | Description |
ram_used | int | N | ram usage percent.[0-100] |
cpu_used | int | N | cpu usage percentage. [0-100] |
power_up_time | date | N | The last power-on time |
cpu_temp | int | N | CPU Temperature: Unit: Celsius |
cpu_temp_unit | string | N | CPU Temperature Unit: Optional values: |
sd_card_used | int | Y | SD Card Usage (Percentage): Range: *Note: If the SD card is not inserted or not formatted, the value is empty. |
Conditions: The request parameters are legal, and the user identity verification is passed.
Status Code: 200 OK
Response Example:
{
"error": 0,
"data": {
"ram_used": 40,
"cpu_used": 30,
"power_up_time": "2022-10-12T02:58:09.989Z",
"cpu_temp": 51,
"cpu_temp_unit": "c",
"sd_card_used" : "12"
},
"message": "success"
}
c. Set the Gateway
Allow authorized users to set the gateway through this interface
- URL:
/open-api/v2/rest/bridge/config
- Method:
PUT
- Header:
- Content-Type: application/json
- Autorization: Bearer <token>
Request parameters:
Attribute | Type | Optional | Description |
volume | int | Y | System volume. [0-100] |
Successful data response:empty Object {}
Conditions: The request parameters are legal, and the user identity verification is passed.
Status Code: 200 OK
Response Example:
{
"error": 0,
"data": {},
"message": "success"
}
d. Get the Gateway info
Allow authorized users to get the gateway info through this interface
- URL:
/open-api/v2/rest/bridge
- Method:
GET
- Header:
- Content-Type: application/json
Request parameters:
Attribute | Type | Optional | Description |
ip | string | N | ip address |
mac | string | N | mac address |
domain | string | Y | Gateway service domain |
fw_version | string | N | Firmware version |
name | string | N | Device name |
Successful data response:empty Object {}
Conditions: None
Status Code: 200 OK
Response Example:
{
"error": 0,
"data": {
"ip": "192.168.31.25",
"mac": "00:0A:02:0B:03:0C",
"domain": "ihost.local",
"fw_version": "1.9.0",
"name": "iHost"
},
"message": "success"
}
e. Gateway Mute
Allows authorized users to mute the gateway through this interface.
- URL:
/open-api/v2/rest/bridge/mute
- Method:
PUT
- Header:
- Content-Type: application/json
- Authorization: Bearer <token>
Request Parameters: none
Successful data response:empty Object {}
Conditions: The request parameters are legal, and the user identity verification is passed.
Status Code: 200 OK
Response Example:
{
"error": 0,
"data": {},
"message": "success"
}
f. Unmute Gateway
Allows authorized users to unmute the gateway through this interface.
- URL:
/open-api/v2/rest/bridge/unmute
- Method:
PUT
- Header:
- Content-Type: application/json
- Authorization: Bearer <token>
Request Parameters: none
Successful data response:empty Object {}
Conditions: The request parameters are legal, and the user identity verification is passed.
Status Code: 200 OK
Response Example:
{
"error": 0,
"data": {},
"message": "success"
}
g. Cancel Gateway Alarm
Allows authorized users to disable the alarm sound status on the gateway through this interface.
- URL:
/open-api/v2/rest/bridge/cancel_alarm
- Method:
PUT
- Header:
- Content-Type: application/json
- Authorization: Bearer <token>
Request Parameters: none
Successful data response:empty Object {}
Conditions: The request parameters are legal, and the user identity verification is passed.
Status Code: 200 OK
Response Example:
{
"error": 0,
"data": {},
"message": "success"
}
3.2 Hardware Function
a. Restart Gateway
Allow authorized user to restart the gateway through this interface
- URL:
/open-api/v2/rest/hardware/reboot
- Method:
POST
- Header:
- Content-Type: application/json
- Autorization: Bearer <token>
Request Parameters: none
Successful data response: empty Object {}
Conditions: The request parameters are legal, and the user identity verification is passed.
Status Code: 200 OK
{
"error": 0,
"data": {},
"message": "success"
}
b. Speaker Control
Allow authorized users to control the speaker through this interface
- URL:
/open-api/v2/rest/hardware/speaker
- Method:
POST
- Header:
- Content-Type: application/json
- Authorization: Bearer <token>
Request parameters:
Attribute | Type | Optional | Description |
type | string | N | Optional parameters: 1.play_sound (play the sound) 2.play_beep (play the beep) |
sound | SoundObject | Y (N if type is play_sound.) | The sound. |
beep | BeepObject | Y (N if type is play_beep.) | The beep |
SoundObject
Attribute | Type | Optional | Description |
name | string | N | The sound name. The supported values can be checked in [Resource List – Supported sound] |
volume | int | N | The sound volume. [0-100] |
countdown | int | N | The duration for the speaker to play the sound, and it will stop playing automatically after the time is up. Unit: second. [0,1799] |
BeepObject
Attribute | Type | Optional | Description |
name | string | N | The deep name. The supported values can be checked in [Resource List – Supported deep] |
volume | int | N | The deep volume. [0-100] |
Successful data response: empty Object {}
Conditions: The request parameters are legal, and the user identity verification is passed.
Status Code: 200 OK
Response Example:
{
"error": 0,
"data": {},
"message": "success"
}
3.3 Device Management Function
a. Supported Device Type
Device Type | Value | iHost Version |
Plug | plug | ≥ 2.1.0 |
Switch | switch | ≥ 2.1.0 |
Light | light | ≥ 2.1.0 |
Curtain | curtain | ≥ 2.1.0 |
Door/Window Sensor | contactSensor | ≥ 2.1.0 |
Motion sensor | motionSensor | ≥ 2.1.0 |
Temperature sensor | temperatureSensor | ≥ 2.1.0 |
Humidity sensor | humiditySensor | ≥ 2.1.0 |
Temperature and humidity sensor | temperatureAndHumiditySensor | ≥ 2.1.0 |
Water leak detector | waterLeakDetector | ≥ 2.1.0 |
Smoke detector | smokeDetector | ≥ 2.1.0 |
Wireless button | button | ≥ 2.1.0 |
Camera | camera | ≥ 2.1.0 |
General sensor | sensor | ≥ 2.1.0 |
Fanlight | fanLight | ≥ 2.1.0 |
AirConditioner | airConditioner | ≥ 2.1.0 |
Fan | fan | ≥ 2.1.0 |
Thermostat | thermostat | ≥ 2.1.0 |
b. Supported Device Capabilities
1. Power Switch (power):
1.1. Capability Declaration Example:
[
{
"capability": "power", // Capability name
"permission": "1100", // ihost does not support configuring soft start/stop, thus no configure field
}
]
1.2. State Attribute:
{
"powerState": "on", // Field powerState indicates the power on/off state. Required. **Type:** string. "on" indicates power on, "off" indicates power off, "toggle" indicates toggle.
}
1.3. Protocol (Query Status & Control Instructions):
Turn On:
{
"power": {
"powerState": "on"
}
}
Turn Off:
{
"power": {
"powerState": "off"
}
}
2. Channel Switch (toggle):
2.1. Capability Declaration Example:
Single Component Example:
[
{
"capability": "toggle", // Capability name
"permission": "1100", // Permission
"name": "1", // Component name, **Type:** String. Optional values: "1" (Channel 1), "2" (Channel 2), "3" (Channel 3), "4" (Channel 4), or other values containing uppercase and lowercase letters and numbers
}
]
Multiple Components Example:
[
{
"capability": "toggle",
"permission": "1100",
"name": "1"
},
{
"capability": "toggle",
"permission": "1100",
"name": "2"
}
]
2.2. State Attribute:
{
"toggleState": "on", // Field toggleState indicates the toggle state of {device_id}'s {name} attribute. Required. **Type:** String. "on" indicates enabled, "off" indicates disabled, "toggle" indicates toggle.
}
2.3. Protocol (Query Status & Control Instructions):
Toggle Format:
{
[capability]: {
[toggle-name]: {
[toggleState]: [value]
}
}
}
Component 1 On, Component 2 Off:
{
"toggle": {
"1": {
"toggleState": "on"
},
"2": {
"toggleState": "off"
}
}
}
3. Channel Inching (toggle-inching):
3.1. Capability Declaration Example:
[
{
"capability": "toggle-inching", // Capability name
"permission": "0010", // Permission
"settings": {
"toggleInchingSetting": {
"permission": "11",
"type": "object",
"value": {
"supported": {
"1": { // Component name
"enable": true, // Whether inching function is enabled. **Type:** Boolean. Required.
"inchingSwitch": "off", // Inching switch setting. **Type:** String. Required. "off" (power off), "on" (power on)
"delay": 1000, // Inching delay time in milliseconds. **Type:** Integer. Required.
"min_delay": 500, // Minimum delay time
"max_delay": 100000 // Maximum delay time
},
"2": { // Component name
"enable": true, // Whether inching function is enabled. **Type:** Boolean. Required.
"inchingSwitch": "off", // Inching switch setting. **Type:** String. Required. "off" (power off), "on" (power on)
"delay": 1000, // Inching delay time in milliseconds. **Type:** Integer. Required.
"min_delay": 500, // Minimum delay time
"max_delay": 100000 // Maximum delay time
},
"3": { // Component name
"enable": true, // Whether inching function is enabled. **Type:** Boolean. Required.
"inchingSwitch": "off", // Inching switch setting. **Type:** String. Required. "off" (power off), "on" (power on)
"delay": 1000, // Inching delay time in milliseconds. **Type:** Integer. Required.
"min_delay": 500, // Minimum delay time
"max_delay": 100000 // Maximum delay time
},
"4": { // Component name
"enable": true, // Whether inching function is enabled. **Type:** Boolean. Required.
"inchingSwitch": "off", // Inching switch setting. **Type:** String. Required. "off" (power off), "on" (power on)
"delay": 1000, // Inching delay time in milliseconds. **Type:** Integer. Required.
"min_delay": 500, // Minimum delay time
"max_delay": 100000 // Maximum delay time
}
}
}
}
}
}
]
3.2. State Attribute
None
3.3. Protocol (Query Status & Control Instructions)
None
4. Brightness Adjustment (brightness):
4.1. Capability Declaration Example:
{
"capability": "brightness", // Capability name
"permission": "1100" // Permission
}
4.2. State Attribute:
{
"brightness": 100, // Field brightness indicates the brightness percentage. Choose between brightness or brightnessDelta. **Type:** Number. Range: 0-100.
}
4.3. Protocol (Query Status & Control Instructions):
Set Brightness to 80%. (0 is darkest and 100 is lightest.)
json
复制代码
{
"brightness": {
"brightness": 80
}
}
5. Color Temperature Adjustment (color-temperature):
5.1. Capability Declaration Example:
{
"capability": "color-temperature", // Capability name
"permission": "1100" // Permission
}
5.2. State Attribute:
{
"colorTemperature": 100, // Field colorTemperature indicates the color temperature percentage. Choose between colorTemperature or colorTemperatureDelta. **Type:** Number. Range: 0-100. 0 indicates warm light, 50 indicates neutral light, 100 indicates cool light.
}
5.3. Protocol (Query Status & Control Instructions):
Adjust Color Temperature to 50%:
json
{
"color-temperature": {
"colorTemperature": 50
}
}
6. Color Adjustment (color-rgb):
6.1. Capability Declaration Example:
{
"capability": "color-rgb", // Capability name
"permission": "1100" // Permission
}
6.2. State Attribute:
{
"red": 255, // Field red represents the red color in the RGB color space. Required. **Type:** Number. Range: 0-255.
"green": 0, // Field green represents the green color in the RGB color space. Required. **Type:** Number. Range: 0-255.
"blue": 255 // Field blue represents the blue color in the RGB color space. Required. **Type:** Number. Range: 0-255.
}
6.3. Protocol (Query Status & Control Instructions):
Set Color to Purple:
{
"color-rgb": {
"red": 255,
"green": 0,
"blue": 255
}
}
7. Percentage Adjustment (percentage):
7.1. Capability Declaration Example:
{
"capability": "percentage",
"permission": "1100",
"settings": { // Optional
"percentageRange": {
"permission": "01",
"type": "numeric",
"min": 0,
"max": 100,
"step": 5
}
}
}
7.2. State Attribute:
{
"percentage": 100, // Field percentage indicates a percentage value. Choose between percentage or percentageDelta. **Type:** Number. Range: 0-100.
}
7.3. Protocol (Query Status & Control Instructions):
Adjust to 40%:
{
"percentage": {
"percentage": 40
}
}
8. Motor Control (motor-control):
8.1. Capability Declaration Example:
{
"capability": "motor-control",
"permission": "1100"
}
8.2. State Attribute:
json
{
"motorControl": "stop", // Field motorControl indicates the motor's state. Required. **Type:** String. Possible values: "open" (open), "close" (close), "stop" (stop), "lock" (lock).
}
8.3. Protocol (Query Status & Control Instructions):
Open Motor:
{
"motor-control": {
"motorControl": "open"
}
}
9. Motor Reversal (motor-reverse):
9.1. Capability Declaration Example:
{
"capability": "motor-reverse",
"permission": "1100"
}
9.2. State Attribute:
{
"motorReverse": true, // Field motorReverse indicates the motor's direction setting. Required. **Type:** Boolean. true indicates forward, false indicates reverse.
}
9.3. Protocol (Query Status & Control Instructions):
Set Motor to Forward:
{
"motor-reverse": {
"motorReverse": true
}
}
10. Motor Calibration (motor-clb)
10.1. Capability Declaration Example:
{
"capability": "motor-clb",
"permission": "0100"
}
10.2. Attributes (State):
{
"motorClb": "calibration" // Field motorClb indicates the calibration status of the motor. Required. **Type:** String. "normal" indicates normal mode (calibrated), "calibration" indicates ongoing calibration.
}
10.3. Protocol (Status Reporting):
Report Motor Status is Being Calibrated:
{
"motor-clb": {
"motorClb": "calibration"
}
}
11. Power On State (startup)
11.1. Capability Declaration Example:
{
"capability": "startup",
"permission": "1100"
}
11.2. Attributes (State):
{
"startup": "on" // Power state upon startup. **Type:** String. Required. Optional values: "on" (power on), "stay" (maintain power), "off" (power off), "toggle" (invert power state).
}
11.3. Protocol (Query Status & Control Instructions):
Set Power State to Always On:
{
"startup": {
"startup": "on"
}
}
12. Wake-up Activation (identify)
12.1. Capability Declaration Example:
{
"capability": "identify",
"permission": "0100"
}
12.2. Attributes (State):
{
"identify": true // Indicates whether the device actively reports recognition results or is activated.
}
12.3. Protocol (Status Reporting & Control Instructions):
Set Wake-up Activation Time:
{
"identify": {
"countdown": 180 // Field countdown indicates the countdown time. Required. **Type:** Number. Unit: seconds.
}
}
Report Activation Status:
{
"identify": {
"identify": true // Indicates whether the device actively reports recognition results or is activated.
}
}
13. Real-time Power Consumption Statistics Switch (power-consumption)
13.1. Capability Declaration Example:
{
"capability": "power-consumption",
"permission": "1101",
"settings": {
"resolution": {
"permission": "01",
"type": "numeric",
"value": 3600
},
"timeZoneOffset": {
"permission": "01",
"type": "numeric",
"min": -12,
"max": 14,
"value": 0
}
}
}
13.2. Attributes (State):
Start/Stop Real-time Statistics:
{
"rlSummarize": true, // Start/stop real-time statistics. **Type:** Boolean. Required.
"timeRange": { // Summarized time range. Required.
"start": "2020-07-05T08:00:00Z", // Start time of power consumption statistics. **Type:** String. Required.
"end": "2020-07-05T09:00:00Z" // End time of power consumption statistics. **Type:** String. Required.
}
}
Query Power Consumption by Time Range:
{
"type": "summarize", // Type of statistics. **Type:** String. Required. Optional values: "rlSummarize" (real-time summary), "summarize" (current summary).
"timeRange": { // Summarized time range. Required if type is "summarize".
"start": "2020-07-05T08:00:00Z", // Start time of power consumption statistics. **Type:** String. Required.
"end": "2020-07-05T09:00:00Z" // End time of power consumption statistics. **Type:** String. Optional. If omitted, indicates current time.
}
}
Respond with Statistics Result within Time Range:
json
{
"type": "summarize", // Type of statistics. **Type:** String. Required. Optional values: "rlSummarize" (real-time summary), "summarize" (current summary).
"rlSummarize": 50.0, // Total power consumption. **Type:** Number. Unit: 0.01 kWh. Optional.
"electricityIntervals": [ // Multiple statistics records divided according to configuration.resolution. Optional. Not present when type is "rlSummarize".
{
"usage": 26.5, // Power consumption. **Type:** Number. Unit: 0.01 kWh. Required.
"start": "2020-07-05T08:00:00Z", // Start time. **Type:** Date. Required.
"end": "2020-07-05T09:00:00Z" // End time. **Type:** Date. Required. If the interval between end and start is smaller than resolution, all reported records are considered invalid.
},
{
"usage": 26.5, // Power consumption. **Type:** Number. Unit: 0.01 kWh. Required.
"start": "2020-07-05T09:00:00Z", // Start time. **Type:** Date. Required.
"end": "2020-07-05T10:00:00Z" // End time. **Type:** Date. Required. If the interval between end and start is smaller than resolution, all reported records are considered invalid.
}
]
}
13.3. Protocol (Status Reporting & Control Instructions):
Start Real-time Statistics:
json
{
"power-consumption": {
"powerConsumption": {
"rlSummarize": true,
"timeRange": {
"start": "2020-07-05T08:00:00Z",
"end": ""
}
}
}
}
Stop Real-time Statistics:
json
{
"power-consumption": {
"powerConsumption": {
"rlSummarize": false,
"timeRange": {
"start": "2020-07-05T08:00:00Z",
"end": "2020-07-05T09:00:00Z"
}
}
}
}
Get Historical Power Consumption:
json
{
"power-consumption": {
"type": "summarize",
"timeRange": {
"start": "2020-07-05T08:00:00Z",
"end": "2020-07-05T09:00:00Z"
}
}
}
Get Real-time Power Consumption:
json
{
"power-consumption": {
"powerConsumption": {
"type": "rlSummarize"
}
}
}
14. Temperature and Humidity Mode Detection (thermostat-mode-detect)
14.1. Capability Declaration Example:
{
"capability": "thermostat-mode-detect",
"permission": "0110",
"name": "temperature", // Type of temperature control detection. **Type:** String. Required. Optional values: "humidity" (humidity detection), "temperature" (temperature detection).
"settings": {
"setpointRange": {
"permission": "11",
"type": "object",
"value": {
"supported": [ // Supported detection settings. Required.
{
"name": "lowerSetpoint", // Detection value should be above this setpoint. At least one of lowerSetpoint or upperSetpoint is required.
"value": { // Detection range. Optional. Specify if preset conditions exist.
"value": 68.0, // Temperature value. **Type:** Number. Required.
"scale": "f" // Temperature unit. Required when name=temperature. Optional values: "c" (Celsius), "f" (Fahrenheit).
}
},
{
"name": "upperSetpoint", // Detection value should be below this setpoint. At least one of lowerSetpoint or upperSetpoint is required.
"value": { // Detection range. Optional. Specify if preset conditions exist.
"value": 68.0, // Temperature value. **Type:** Number. Required.
"scale": "f" // Temperature unit. Required when name=temperature. Optional values: "c" (Celsius), "f" (Fahrenheit).
}
}
]
}
},
"supportedModes": {
"type": "enum",
"permission": "01",
"values": [
"COMFORT",
"COLD",
"HOT"
]
}
}
}
{
"capability": "thermostat-mode-detect",
"permission": "0110",
"name": "humidity", // Type of temperature control detection. **Type:** String. Required. Optional values: "humidity" (humidity detection), "temperature" (temperature detection).
"settings": {
"setpointRange": {
"permission": "11",
"type": "object",
"value": {
"supported": [ // Supported detection settings. Required.
{
"name": "lowerSetpoint", // Detection value should be above this setpoint. At least one of lowerSetpoint or upperSetpoint is required.
"value": { // Detection range. Optional. Specify if preset conditions exist.
"value": 68.0, // Humidity value. **Type:** Number. Required
}
},
{
"name": "upperSetpoint", // Detection value should be below this setpoint. At least one of lowerSetpoint or upperSetpoint is required.
"value": { // Detection range. Optional. Specify if preset conditions exist.
"value": 68.0, // Humidity value. **Type:** Number. Required
}
}
]
}
},
"supportedModes": {
"type": "enum",
"permission": "01",
"values": [
"COMFORT",
"COLD",
"HOT"
]
}
}
}
14.2. Attributes (State):
{
"mode": "COMFORT" // Mode ID. Optional. Supported values: "COMFORT" (Comfort Mode), "COLD" (Cold Mode), "HOT" (Hot Mode), "DRY" (Dry Mode), "WET" (Wet Mode).
}
14.3. Protocol (Query Status & Control Instructions):
Format:
{
[capability] :{
[mode name] :{
"mode": [value]
}
}
}
Example:
{
"thermostat-mode-detect": {
"humidity": {
"mode": "COMFORT"
}
}
}
15. Thermostat Mode (thermostat-mode)
15.1. Capability Declaration Example:
{
"capability": "thermostat",
"permission": "1100",
"name": "thermostat-mode",
"settings": {
"supportedModes": {
"type": "enum",
"permission": "01",
"values": [
"MANUAL",
"AUTO",
"ECO"
]
}
}
}
15.2. Attributes (State):
{
"thermostatMode": "MANUAL" // Thermostat operating mode. **Type:** String. Optional values: "MANUAL", "AUTO", "ECO".
}
15.3. Protocol (Query Status & Control Instructions):
{
"thermostat": {
"thermostat-mode": {
"thermostatMode": "MANUAL"
}
}
}
16. Thermostat Adaptive Recovery Status (thermostat/adaptive-recovery-status)
16.1. Capability Declaration Example:
{
"capability": "thermostat",
"permission": "0100",
"name": "adaptive-recovery-status" // Thermostat adaptive recovery status
}
16.2. Attributes (State):
{
"adaptiveRecoveryStatus": "HEATING" // Thermostat adaptive recovery status. **Type:** String. Optional values: "HEATING", "INACTIVE".
}
16.3. Protocol (Query Status & Control Instructions):
{
"thermostat": {
"adaptive-recovery-status": {
"adaptiveRecoveryStatus": "HEATING"
}
}
}
17. Thermostat Mode Target Temperature Setting (thermostat-target-setpoint)
17.1. Capability Declaration Example:
[[
{
"capability": "thermostat-target-setpoint",
"name": "manual-mode",
"permission": "1110",
"settings": {
"temperatureUnit":{
"type": "enum",
"permission": "11",
"value": "c",
"values": [
"c",
"f"
]
},
"temperatureRange":{
"type": "numeric",
"permission": "01",
"min": 4,
"max": 35,
"step": 0.5
}
}
},
{
"capability": "thermostat-target-setpoint",
"name": "eco-mode",
"permission": "1110",
"settings": {
"temperatureUnit":{
"type": "enum",
"permission": "11",
"value": "c",
"values": [
"c",
"f"
]
},
"temperatureRange":{
"type": "numeric",
"permission": "01",
"min": 4,
"max": 35,
"step": 0.5
}
}
},
{
"capability": "thermostat-target-setpoint",
"name": "auto-mode",
"permission": "0110",
"settings": {
"temperatureUnit":{
"type": "enum",
"permission": "11",
"value": "c",
"values": [
"c",
"f"
]
},
"temperatureRange":{
"type": "numeric",
"permission": "01",
"min": 4,
"max": 35,
"step": 0.5
},
"weeklySchedule":{
"type": "object",
"permission": "11",
"value": {
"maxEntryPerDay": 2,
"Monday": [
{
"startTimeInMinutes": 440, // Start time in minutes. **Type:** int. E.g., 7:20 AM = 440 minutes.
"upperSetpoint": 36.5, // Maximum target temperature. **Type:** number.
"lowerSetpoint": 20 // Minimum target temperature. **Type:** number.
},
{
"startTimeInMinutes": 900, // Start time in minutes. E.g., 3:00 PM = 900 minutes.
"upperSetpoint": 26.5, // Maximum target temperature. **Type:** number.
"lowerSetpoint": 21 // Minimum target temperature. **Type:** number.
}
],
"Tuesday": [...
],
"Wednesday": [...
],
"Thursday": [...
],
"Friday": [...
],
"Saturday": [...
],
"Sunday": [...
],
}
}
}
}
]
17.2. Attributes (State):
{
"targetSetpoint": 30 // Target temperature for the specified mode. **Type:** number, in the unit specified by temperatureUnit.
}
17.3. Protocol (Query Status & Control Instructions):
{
"thermostat-target-setpoint": {
"manual-mode": {
"targetSetpoint": 30
},
"auto-mode": {
"targetSetpoint": 30
}
}
}
18. Sensor Detection (detect)
18.1. Capability Declaration Example:
{
"capability": "detect",
"permission": "0110",
"settings": { // Optional
"detectInterval": {
"permission": "11",
"type": "numeric",
"value": 300
},
"detectSensitivity": {
"permission": "11",
"type": "numeric",
"value": 1000
}
}
}
18.2. Attributes (State):
{
"detected": true // Detection result. **Type:** Boolean. `true` indicates detection, `false` indicates no detection.
}
18.3. Protocol (Query Status & Control Instructions):
{
"detect": {
"detected": true
}
}
19. Temperature Detection (temperature)
19.1. Capability Declaration Example:
{
"capability": "temperature",
"permission": "0110",
"settings": { // Optional
"temperatureCalibration": { // Optional temperature calibration
"type": "numeric",
"permission": "11",
"min": -7, // Minimum value
"max": 7, // Maximum value
"step": 0.2, // Temperature adjustment step, unit same as temperatureUnit
"value": 5.2 // Current temperature calibration value. **Type:** number.
},
"temperatureUnit": { // Optional temperature unit
"type": "enum",
"permission": "11",
"value": "c",
"values": [
"c",
"f"
]
},
"temperatureRange": { // Optional temperature detection range
"type": "numeric",
"permission": "01",
"min": -40,
"max": 80
}
}
}
19.2. Attributes (State):
json
复制代码
{
"temperature": 26.5 // Current temperature. **Type:** Number, in Celsius.
}
19.3. Protocol (Query Status & Control Instructions):
{
"temperature": {
"temperature": 20
}
}
20. Humidity Detection (humidity)
20.1. Capability Declaration Example:
{
"capability": "humidity",
"permission": "0100",
"settings": { // Optional humidity detection range.
"humidityRange": {
"type": "numeric",
"permission": "01",
"min": 0,
"max": 100
}
}
}
20.2. Attributes (State):
{
"humidity": 50 // Current relative humidity (percentage). **Type:** Number, range 0-100.
}
20.3. Protocol (Query Status & Control Instructions):
{
"humidity": {
"humidity": 20
}
}
21. Battery Detection (battery)
21.1. Capability Declaration Example:
{
"capability": "battery",
"permission": "0100"
}
21.2. Attributes (State):
{
"battery": 95 // Remaining battery percentage. **Type:** Number, range -1 to 100. Note: -1 indicates unknown battery level.
}
21.3. Protocol (Query Status & Control Instructions):
{
"battery": {
"battery": 40
}
}
22. Single Button Detection (press)
22.1. Capability Declaration Example:
{
"capability": "press",
"permission": "1100",
"settings": { // Optional
"actions": { // Button actions, optional.
"type": "enum",
"permission": "01",
"values": [ // Optional button actions. Default values are "singlePress", "doublePress", "longPress". Configured actions replace defaults.
]
}
}
}
22.2. Attributes (State):
{
"press": "singlePress" // Button press type. **Type:** String. Standard values: "singlePress" (single or short press), "doublePress" (double press), "longPress" (long press).
}
22.3. Protocol (Query Status & Control Instructions):
{
"press": {
"press": "singlePress"
}
}
23. Multi-Button Detection (multi-press)
23.1. Capability Declaration Example:
{
"capability": "multi-press",
"permission": "0100",
"name": "1", // Name of the multi-press attribute. **Type:** String. Only alphanumeric characters allowed.
"settings": { // Optional
"actions": { // Button actions, optional.
"type": "enum",
"permission": "01",
"values": [ // Optional button actions. Default values are "singlePress", "doublePress", "longPress". Configured actions replace defaults.
]
}
}
}
23.2. Attributes (State):
{
"press": "singlePress" // Button press type. **Type:** String. Standard values: "singlePress" (single or short press), "doublePress" (double press), "longPress" (long press).
}
23.3. Protocol (Query Status & Control Instructions):
{
[capability] :{
[multi-press-name]:{
press:[value]
}
}
}
example:
{
"multi-press": {
"1": {
"press": "singlePress"
},
"2": {
"press": "doublePress"
}
}
}
24. Signal Strength Detection (rssi)
24.1. Capability Declaration Example:
{
"capability": "rssi",
"permission": "0100"
}
24.2. Attributes (State):
{
"rssi": -65 // Wireless signal strength (Received Signal Strength Indicator). **Type:** Number, unit dBm, negative integer values.
}
24.3. Protocol (Query Status & Control Instructions):
{
"rssi": {
"rssi": -65
}
}
25. Tamper Detection (tamper-alert)
25.1. Capability Declaration Example:
{
"capability": "tamper-alert",
"permission": "0100"
}
25.2. Attributes (State):
{
"tamper": "clear" // Tamper status. **Type:** String. Possible values: "clear" (not tampered), "detected" (tampered).
}
25.3. Protocol (Query Status & Control Instructions):
{
"tamper-alert": {
"tamper": "clear" // Tamper status. **Type:** String. Possible values: "clear" (not tampered), "detected" (tampered).
}
}
26. Illumination Level Detection (illumination-level)
26.1. Capability Declaration Example:
{
"capability": "illumination-level",
"permission": "0100"
}
26.2. Attributes (State):
{
"level": "brighter" // Brightness level. **Type:** String. Possible values: "brighter" (brighter), "darker" (darker).
}
26.3. Protocol (Query Status & Control Instructions):
{
"illumination-level": {
"level": "brighter"
}
}
27. Voltage Detection (voltage)
27.1. Capability Declaration Example:
{
"capability": "voltage",
"permission": "0100"
}
27.2. Attributes (State):
{
"voltage": 50 // Current voltage value, in 0.01V units. **Type:** Number, non-negative values.
}
27.3. Protocol (Query Status & Control Instructions):
{
"voltage": {
"voltage": 50
}
}
28. Electric Current Detection (electric-current)
28.1. Capability Declaration Example:
{
"capability": "electric-current",
"permission": "0100"
}
28.2. Attributes (State):
{
"electric-current": 50 // Current current value, in 0.01A units. **Type:** Number, non-negative integer values.
}
28.3. Protocol (Query Status & Control Instructions):
{
"electric-current": {
"electric-current": 50
}
}
29. Electric Power Detection (electric-power)
29.1. Capability Declaration Example:
{
"capability": "electric-power",
"permission": "0100"
}
29.2. Attributes (State):
{
"electric-power": 50 // Current power value, in 0.01W units. **Type:** Number, non-negative integer values.
}
29.3. Protocol (Query Status & Control Instructions):
{
"electric-power": {
"electric-power": 50
}
}
30. Fault Detection (fault)
30.1. Capability Declaration Example:
{
"capability": "fault",
"permission": "0100"
}
30.2. Attributes (State):
{
"fault": "none" // Fault status. **Type:** String. Possible values: "none" (no fault), "detected" (fault detected).
}
30.3. Protocol (Query Status & Control Instructions):
{
"fault": {
"fault": "none"
}
}
31. Threshold Breaker (threshold-breaker)
31.1. Capability Declaration Example:
{
"capability": "threshold-breaker",
"permission": "0010",
"settings": {
"supportedSetting": {
"type": "object",
"permission": "11",
"value": {
"supported": [
{
"name": "lowerPower", // Low power threshold breaker
"value": {
"value": 50, // Detection power value, in 0.01W units. **Type:** Integer. Range: >=0.
"min_range": 10, // Minimum detection power value, in 0.01W units. **Type:** Integer. Range: >=0.
"max_range": 3500 // Maximum detection power value, in 0.01W units. **Type:** Integer. Range: >=0.
}
},
{
"name": "upperPower", // High power threshold breaker
"value": {
"value": 50, // Detection power value, in 0.01W units. **Type:** Integer. Range: >=0.
"min_range": 10, // Minimum detection power value, in 0.01W units. **Type:** Integer. Range: >=0.
"max_range": 3500 // Maximum detection power value, in 0.01W units. **Type:** Integer. Range: >=0.
}
},
{
"name": "lowerElectricCurrent", // Low current threshold breaker
"value": {
"value": 50, // Detection current value, in 0.01A units. **Type:** Integer. Range: >=0.
"min_range": 10, // Minimum detection current value, in 0.01A units. **Type:** Integer. Range: >=0.
"max_range": 1500 // Maximum detection current value, in 0.01A units. **Type:** Integer. Range: >=0.
}
},
{
"name": "upperElectricCurrent", // High current threshold breaker
"value": {
"value": 50, // Detection current value, in 0.01A units. **Type:** Integer. Range: >=0.
"min_range": 10, // Minimum detection current value, in 0.01A units. **Type:** Integer. Range: >=0.
"max_range": 1500 // Maximum detection current value, in 0.01A units. **Type:** Integer. Range: >=0.
}
},
{
"name": "lowerVoltage", // Low voltage threshold breaker
"value": {
"value": 50, // Detection voltage value, in 0.01V units. **Type:** Integer. Range: >=0.
"min_range": 10, // Minimum detection voltage value, in 0.01V units. **Type:** Integer. Range: >=0.
"max_range": 24000 // Maximum detection voltage value, in 0.01V units. **Type:** Integer. Range: >=0.
}
},
{
"name": "upperVoltage", // High voltage threshold breaker
"value": {
"value": 50, // Detection voltage value, in 0.01V units. **Type:** Integer. Range: >=0.
"min_range": 10, // Minimum detection voltage value, in 0.01V units. **Type:** Integer. Range: >=0.
"max_range": 24000 // Maximum detection voltage value, in 0.01V units. **Type:** Integer. Range: >=0.
}
}
]
}
}
}
}
31.2. Attributes (State)
- None
31.3. Protocol (Query Status & Control Instructions)
- None
32. Inch Function (inching)
32.1. Capability Declaration Example:
{
"capability": "inching",
"permission": "0010",
"settings": {
"inchingEnable": { // Inch function enable setting
"permission": "11",
"type": "boolean",
"value": false
},
"inchingSwitch": { // Inch action setting
"type": "enum",
"permission": "11",
"value": "on",
"values": ["on", "off"]
},
"inchingDelay": { // Inch time setting
"type": "numeric",
"permission": "11",
"min": 500,
"max": 100000,
"value": 1000,
"unit": "ms"
}
}
}
32.2. Attributes (State):
- None
32.3. Protocol (Query Status & Control Instructions):
- None
33. Camera Stream (camera-stream)
33.1. Capability Declaration Example:
{
"capability": "camera-stream",
"permission": "0010",
"settings": {
"streamSetting": {
"type": "object",
"permission": "11",
"value": {
"username": "String", // Access account. **Type:** String. Required.
"password": "String", // Access password. **Type:** String. Required.
"streamUrl": "rtsp://<username>:<password>@<hostname>:<port>/streaming/channel01", // Stream URL. **Type:** String. Required.
"videoCodec": "", // Video codec. **Type:** String. Required. Optional parameters to be defined.
"resolution": { // Video resolution. Required.
"width": 1080, // Width. **Type:** Number. Required.
"height": 720 // Height. **Type:** Number. Required.
},
"keyFrameInterval": 5, // Key frame interval. **Type:** String. Required.
"audioCodec": "G711", // Audio codec. **Type:** String. Required. Optional parameters to be defined.
"samplingRate": 50, // Audio sampling rate, in Hz. **Type:** Number. Required. Optional parameters to be defined.
"dataRate": 50 // Bitrate. **Type:** Number. Required. Unit: kb/s.
}
}
}
}
33.2. Attributes (State):
- None
33.3. Protocol (Query Status & Control Instructions):
- None
34. Mode Control (mode)
34.1. Capability Declaration Example:
[
{
"capability": "mode",
"name": "fanLevel",
"permission": "1100",
"settings": {
"supportedValues": {
"type": "enum",
"permission": "01",
"values": ["low", "medium", "high"] // Custom mode values. Configured values override defaults.
}
}
},
{
"capability": "mode",
"name": "thermostatMode",
"permission": "1100",
"settings": {
"supportedValues": {
"type": "enum",
"permission": "01",
"values": ["auto", "manual"] // Custom mode values. Configured values override defaults.
}
}
},
{
"capability": "mode",
"name": "airConditionerMode",
"permission": "1100",
"settings": {
"supportedValues": {
"type": "enum",
"permission": "01",
"values": ["cool", "heat", "auto", "fan", "dry"] // Custom mode values. Configured values override defaults.
}
}
},
{
"capability": "mode",
"name": "fanMode",
"permission": "1100",
"settings": {
"supportedValues": {
"type": "enum",
"permission": "01",
"values": ["normal", "sleep", "child"] // Custom mode values. Configured values override defaults.
}
}
},
{
"capability": "mode",
"name": "horizontalAngle",
"permission": "1100",
"settings": {
"supportedValues": {
"type": "enum",
"permission": "01",
"values": ["30", "60", "90", "120", "180", "360"] // Custom mode values. Configured values override defaults.
}
}
},
{
"capability": "mode",
"name": "verticalAngle",
"permission": "1100",
"settings": {
"supportedValues": {
"type": "enum",
"permission": "01",
"values": ["30", "60", "90", "120", "180", "360"] // Custom mode values. Configured values override defaults.
}
}
}
]
34.2. Attributes (State):
{
"modeValue": "low" // Mode value. **Type:** String. Required. Refer to supported preset modes.
}
34.3. Protocol (Query Status & Control Instructions):
{
"mode": {
"fanLevel": {
"modeValue": "low"
}
}
}
35. Carbon Dioxide Detection (co2)
35.1. Capability Declaration Example:
{
"capability": "co2",
"permission": "0100",
"settings": {
"co2Range": {
"type": "numeric",
"permission": "01",
"min": 400,
"max": 10000
}
}
}
35.2. Attributes (State):
{
"co2": 111 // Carbon dioxide concentration. **Type:** Integer. Unit: ppm.
}
35.3. Protocol (Query Status & Control Instructions):
{
"co2": {
"co2": 500
}
}
36. Illumination Detection (illumination)
36.1. Capability Declaration Example:
{
"capability": "illumination",
"permission": "0100",
"settings": {
"illuminationRange": {
"type": "numeric",
"permission": "01",
"min": 0,
"max": 160000
}
}
}
36.2. Attributes (State):
{
"illumination": 11 // Illumination level. **Type:** Integer. Unit: lux.
}
36.3. Protocol (Query Status & Control Instructions):
{
"illumination": {
"illumination": 50
}
}
37. Smoke Detection (smoke)
37.1. Capability Declaration Example:
{
"capability": "smoke",
"permission": "0100"
}
37.2. Attributes (State):
{
"smoke": true // Detection result. **Type:** Boolean. `true` indicates detection, `false` indicates no detection.
}
37.3. Protocol (Query Status & Control Instructions):
{
"smoke": {
"smoke": true
}
}
38. Door Magnet Open/Close Detection (contact)
38.1. Capability Declaration Example:
{
"capability": "contact",
"permission": "0100"
}
38.2. Attributes (State):
{
"contact": true // Detection result. **Type:** Boolean. `true` indicates detection, `false` indicates no detection.
}
38.3. Protocol (Query Status & Control Instructions):
{
"contact": {
"contact": true
}
}
39. Motion Detection (motion)
39.1. Capability Declaration Example:
{
"capability": "motion",
"permission": "0110",
"settings": {
"motionInterval": {
"type": "numeric",
"permission": "11",
"value": 300
},
"motionSensitivity": {
"type": "numeric",
"permission": "11",
"value": 1000
}
}
}
39.2. Attributes (State):
{
"motion": true // Detection result. **Type:** Boolean. `true` indicates detection, `false` indicates no detection.
}
39.3. Protocol (Query Status & Control Instructions):
{
"motion": {
"motion": true
}
}
40. Water Leak Detection (water-leak)
40.1. Capability Declaration Example:
{
"capability": "water-leak",
"permission": "0100"
}
40.2. Attributes (State):
{
"waterLeak": true // Field `waterLeak` indicates the current detection result. **Type:** Boolean. `true` indicates detection, `false` indicates no detection.
}
40.3. Protocol (Query Status & Control Instructions):
{
"water-leak": {
"waterLeak": true
}
}
41. Window Detection Switch (window-detection)
41.1. Capability Declaration Example:
{
"capability": "window-detection",
"permission": "1100"
}
41.2. Attributes (State):
{
"powerState": "on" // Field `powerState` indicates the power state. **Type:** String. `on` indicates power is on, `off` indicates power is off.
}
41.3. Protocol (Query Status & Control Instructions):
{
"window-detection": {
"powerState": "on"
}
}
42. Child Lock Switch (child-lock)
42.1. Capability Declaration Example:
{
"capability": "child-lock",
"permission": "1100"
}
42.2. Attributes (State):
{
"powerState": "on" // Field `powerState` indicates the power state. **Type:** String. `on` indicates power is on, `off` indicates power is off.
}
42.3. Protocol (Query Status & Control Instructions):
{
"child-lock": {
"powerState": "on"
}
}
43. Anti-Direct Blow Switch (anti-direct-blow)
43.1. Capability Declaration Example:
{
"capability": "anti-direct-blow",
"permission": "1100"
}
43.2. Attributes (State):
{
"powerState": "on" // Field `powerState` indicates the power state. **Type:** String. `on` indicates power is on, `off` indicates power is off.
}
43.3. Protocol (Query Status & Control Instructions):
{
"anti-direct-blow": {
"powerState": "on"
}
}
44. Horizontal Swing Switch (horizontal-swing)
44.1. Capability Declaration Example:
{
"capability": "horizontal-swing",
"permission": "1100"
}
44.2. Attributes (State):
{
"powerState": "on" // Field `powerState` indicates the power state. **Type:** String. `on` indicates power is on, `off` indicates power is off.
}
44.3. Protocol (Query Status & Control Instructions):
{
"horizontal-swing": {
"powerState": "on"
}
}
45. Vertical Swing Switch (vertical-swing)
45.1. Capability Declaration Example:
{
"capability": "vertical-swing",
"permission": "1100"
}
45.2. Attributes (State):
{
"powerState": "on" // Field `powerState` indicates the power state. **Type:** String. `on` indicates power is on, `off` indicates power is off.
}
45.3. Protocol (Query Status & Control Instructions):
{
"vertical-swing": {
"powerState": "on"
}
}
46. ECO Mode Switch (eco)
46.1. Capability Declaration Example:
{
"capability": "eco",
"permission": "1100"
}
46.2. Attributes (State):
{
"powerState": "on" // Field `powerState` indicates the power state. **Type:** String. `on` indicates power is on, `off` indicates power is off.
}
46.3. Protocol (Query Status & Control Instructions):
{
"eco": {
"powerState": "on"
}
}
47. Toggle Startup (toggle-startup)
47.1. Capability Declaration Example:
{
"capability": "toggle-startup",
"permission": "1100",
"name": "1" // Field `name` specifies the attribute name for `toggle-startup`. **Type:** String. Only letters and numbers are allowed.
}
47.2. Attributes (State):
{
"startup": "on" // **Type:** String. Options: `on` (power on), `stay` (power hold), `off` (power off).
}
47.3. Protocol (Query Status & Control Instructions):
{
"toggle-startup": {
"1": {
"startup": "on"
},
"2": {
"startup": "off"
}
}
}
48. Detect Hold (detect-hold)
48.1. Capability Declaration Example:
{
"capability": "detect-hold",
"permission": "0100",
"settings": {
"detectHoldEnable": { // Detect hold enable setting
"permission": "01",
"type": "boolean",
"value": false
},
"detectHoldSwitch": { // Detect hold action setting
"type": "enum",
"permission": "01",
"value": "on",
"values": ["on", "off"]
},
"detectHoldTime": { // Detect hold time setting
"type": "numeric",
"permission": "01",
"min": 1,
"max": 359,
"value": 5,
"unit": "minute"
}
}
}
48.2. Attributes (State):
{
"detectHold": "on" // Field `detectHold` indicates the state detection hold status. **Type:** String. `on` means detection hold is active, `off` means detection hold is inactive.
}
48.3. Protocol (Query Status & Control Instructions):
{
"detect-hold": {
"detectHold": "on"
}
}
49. Toggle Identify/Activate (toggle-identify)
49.1. Capability Declaration Example:
{
"capability": "toggle-identify",
"permission": "1100", // Note: This capability is not used for reporting in the current version (V1.13.7) on ihost.
"name": "1" // Component name. **Type:** String. Optional values: "1" (Channel 1), "2" (Channel 2), "3" (Channel 3), "4" (Channel 4).
}
49.2. Attributes (State):
{
"identify": true, // Indicates the device has actively reported identification or activation.
"countdown": 180 // Field `countdown` indicates activation duration. **Type:** Number. Unit: seconds.
}
49.3. Protocol (Query Status & Control Instructions):
{
"toggle-identify": {
"1": {
"countdown": 180 // Activate device for 180 seconds.
}
}
}
// Device reports self-activation
{
"toggle-identify": {
"1": {
"identify": true
}
}
}
50. Toggle Voltage Detection (toggle-voltage)
50.1. Capability Declaration Example:
{
"capability": "toggle-voltage",
"permission": "1100"
}
50.2. Attributes (State):
{
"voltage": 230 // Field `voltage` indicates the detected voltage. **Type:** Number. Unit: Volts.
}
50.3. Protocol (Query Status & Control Instructions):
{
"toggle-voltage": {
"voltage": 230
}
}
51. Subcomponent Electric Current Detection (toggle-electric-current)
51.1. Capability Declaration Example:
[
{
"capability": "toggle-electric-current",
"permission": "0100",
"name": "1" // Component name. **Type:** String. Optional values: "1" (Channel 1), "2" (Channel 2), "3" (Channel 3), "4" (Channel 4).
}
]
51.2. Attributes (State):
{
"electricCurrent": 50 // Field `electricCurrent` represents the current value. **Unit:** 0.01 A. **Type:** Integer. Value must be greater than or equal to 0.
}
51.3. Protocol (Query Status & Control Instructions):
{
"toggle-electric-current": {
"1": {
"electricCurrent": 50
}
}
}
52. Subcomponent Power Detection (toggle-electric-power)
52.1. Capability Declaration Example:
[
{
"capability": "toggle-electric-power",
"permission": "0100",
"name": "1" // Component name. **Type:** String. Optional values: "1" (Channel 1), "2" (Channel 2), "3" (Channel 3), "4" (Channel 4).
}
]
52.2. Attributes (State):
{
"electricPower": 50, // Field `electricPower` represents the current power value. **Unit:** 0.01 W. **Type:** Integer. Value must be greater than or equal to 0.
"reactivePower": 50, // Field `reactivePower` represents the current reactive power value. **Unit:** 0.01 W. **Type:** Integer. Optional.
"activePower": 50, // Field `activePower` represents the current active power value. **Unit:** 0.01 W. **Type:** Integer. Optional.
"apparentPower": 50 // Field `apparentPower` represents the current apparent power value. **Unit:** 0.01 W. **Type:** Integer. Optional.
}
52.3. Protocol (Query Status & Control Instructions):
{
"toggle-electric-power": {
"1": {
"electricPower": 50,
"reactivePower": 50,
"activePower": 50,
"apparentPower": 50
}
}
}
53. Subcomponent Power Consumption Statistics (toggle-power-consumption)
53.1. Capability Declaration Example:
[
{
"capability": "toggle-power-consumption",
"permission": "1101",
"name": "1", // Component name. **Type:** String. Optional values: "1" (Channel 1), "2" (Channel 2), "3" (Channel 3), "4" (Channel 4).
"settings": {
"resolution": {
"permission": "01",
"type": "numeric",
"value": 3600
},
"timeZoneOffset": {
"permission": "01",
"type": "numeric",
"min": -12,
"max": 14,
"value": 0
}
}
}
]
53.2. Attributes (State):
Start/Stop Real-time Statistics:
{
"rlSummarize": true, // Start or stop real-time statistics. **Type:** Boolean. Required.
"timeRange": { // Summary time range. Required.
"start": "2020-07-05T08:00:00Z", // Start time of the power consumption. **Type:** String. Required.
"end": "2020-07-05T09:00:00Z" // End time of the power consumption. **Type:** String. Required.
}
}
Query Power Consumption by Time Range:
{
"type": "summarize", // Summary type. **Type:** String. Required. Options: "rlSummarize" (Real-time summary), "summarize" (Current summary).
"timeRange": { // Summary time range, required when type is "summarize".
"start": "2020-07-05T08:00:00Z", // Start time of the power consumption. **Type:** String. Required.
"end": "2020-07-05T09:00:00Z" // End time of the power consumption. **Type:** String. Optional. If not provided, defaults to the current time.
}
}
Response for Power Consumption within Time Range:
{
"type": "summarize", // Summary type. **Type:** String. Required.
"rlSummarize": 50.0, // Total power consumption. **Unit:** 0.01 kWh. **Type:** Number. Optional.
"electricityIntervals": [ // Divided by `configuration.resolution` into multiple records. Optional. Not present when type is "rlSummarize".
{
"usage": 26.5, // Power consumption. **Unit:** 0.01 kWh. **Type:** Number. Required.
"start": "2020-07-05T08:00:00Z", // Start time. **Type:** String. Required.
"end": "2020-07-05T09:00:00Z" // End time. **Type:** String. Required. Records with intervals shorter than resolution are considered invalid.
},
{
"usage": 26.5, // Power consumption. **Unit:** 0.01 kWh. **Type:** Number. Required.
"start": "2020-07-05T09:00:00Z", // Start time. **Type:** String. Required.
"end": "2020-07-05T10:00:00Z" // End time. **Type:** String. Required. Records with intervals shorter than resolution are considered invalid.
}
]
}
53.3. Protocol (Query Status & Control Instructions):
Start Real-time Statistics:
{
"toggle-power-consumption": {
"1": {
"rlSummarize": true,
"timeRange": {
"start": "2020-07-05T08:00:00Z",
"end": ""
}
}
}
}
Stop Real-time Statistics:
{
"toggle-power-consumption": {
"1": {
"rlSummarize": false,
"timeRange": {
"start": "2020-07-05T08:00:00Z",
"end": "2020-07-05T09:00:00Z"
}
}
}
}
Get Historical Power Consumption:
{
"toggle-power-consumption": {
"1": {
"type": "summarize",
"timeRange": {
"start": "2020-07-05T08:00:00Z",
"end": "2020-07-05T09:00:00Z"
}
}
}
}
Get Real-time Power Consumption:
{
"toggle-power-consumption": {
"1": {
"type": "rlSummarize"
}
}
}
54. Link Quality Indicator (lqi)
54.1. Capability Declaration Example:
{
"capability": "lqi",
"permission": "0100"
}
54.2. Attributes (State):
{
"lqi": 60 // Field `lqi` represents the link quality indicator. **Type:** Number. Value range: 0 to 255.
}
54.3. Protocol (Query Status & Control Instructions):
{
"lqi": {
"lqi": 60
}
}
55. Functional Configuration (configuration)
55.1. Capability Declaration Example:
[
{
"capability": "configuration",
"permission": "1100"
}
]
55.2. Attributes (State):
{
"deviceConfiguration": { // Device-related functional configuration
"defaultResolution": 300, // Default resolution for reading moisture saturation data. **Type:** Integer. **Unit:** Seconds. Required.
"maxResolution": 86400, // Maximum resolution for reading moisture saturation data. **Type:** Integer. **Unit:** Seconds. Required.
"minResolution": 60 // Minimum resolution for reading moisture saturation data. **Type:** Integer. **Unit:** Seconds. Required.
}
}
55.3. Protocol (Query Status & Control Instructions):
{
"configuration": {
"deviceConfiguration": { // Device-related functional configuration
"defaultResolution": 300, // Default resolution for reading moisture saturation data. **Type:** Integer. **Unit:** Seconds. Required.
"maxResolution": 86400, // Maximum resolution for reading moisture saturation data. **Type:** Integer. **Unit:** Seconds. Required.
"minResolution": 60 // Minimum resolution for reading moisture saturation data. **Type:** Integer. **Unit:** Seconds. Required.
}
}
}
56. System (system)
56.1. Capability Declaration Example:
[
{
"capability": "system",
"permission": "1000"
}
]
56.2. Attributes (State):
{
"restart": true // Restart device command. **Type:** Boolean. Required. Value must be `true`.
}
56.3. Protocol (Query Status & Control Instructions):
{
"system": { // Capability-related configuration. Optional.
"restart": true
}
}
57. Moisture (moisture)
57.1. Capability Declaration Example:
[
{
"capability": "moisture",
"permission": "0100"
}
]
57.2. Attributes (State):
{
"moisture": 55.5 // Field `moisture` represents the moisture saturation percentage. **Type:** Number. Required. Range: 0 to 100.
}
57.3. Protocol (Query Status & Control Instructions):
{
"moisture": {
"moisture": 55.5
}
}
58. Barometric Pressure (barometric-pressure)
58.1. Capability Declaration Example:
[
{
"capability": "barometric-pressure",
"permission": "0100",
"settings": {
"barometricPressureRange": {
"type": "numeric",
"permission": "01",
"min": 540, // Minimum value. **Type:** Integer. Required. Must be less than `max`.
"max": 1100 // Maximum value. **Type:** Integer. Required. Must be greater than `min`.
}
}
}
]
58.2. Attributes (State):
{
"barometricPressure": 50 // Barometric pressure value. **Type:** Integer. Required. **Unit:** hPa.
}
58.3. Protocol (Query Status & Control Instructions):
{
"barometric-pressure": {
"barometricPressure": 50
}
}
59. Wind Speed (wind-speed)
59.1. Capability Declaration Example:
[
{
"capability": "wind-speed",
"permission": "0100",
"settings": {
"windSpeedRange": {
"type": "numeric",
"permission": "01",
"min": 0, // Minimum value. **Type:** Number. Required. Must be less than `max`.
"max": 50 // Maximum value. **Type:** Number. Required. Must be greater than `min`.
}
}
}
]
59.2. Attributes (State):
{
"windSpeed": 50 // Wind speed value. **Type:** Number. Required. **Unit:** m/s (meters per second).
}
59.3. Protocol (Query Status & Control Instructions):
{
"wind-speed": {
"windSpeed": 50
}
}
60. Wind Direction (wind-direction)
60.1. Capability Declaration Example:
[
{
"capability": "wind-direction",
"permission": "0100"
}
]
60.2. Attributes (State):
{
"windDirection": 10 // Wind direction. **Type:** Integer. Required. **Unit:** Degrees. Range: 0 to 360 degrees (clockwise direction).
}
60.3. Protocol (Query Status & Control Instructions):
{
"wind-direction": {
"windDirection": 10
}
}
61. Rainfall (rainfall)
61.1. Capability Declaration Example:
[
{
"capability": "rainfall",
"permission": "0100",
"settings": {
"rainfallRange": {
"type": "numeric",
"permission": "01",
"min": 0, // Minimum value. **Type:** Number. Required. Must be less than `max`.
"max": 450 // Maximum value. **Type:** Number. Required. Must be greater than `min`.
}
}
}
]
61.2. Attributes (State):
{
"rainfall": 11.11 // Rainfall value. **Type:** Number. Required. **Unit:** mm/h (millimeters per hour).
}
61.3. Protocol (Query Status & Control Instructions):
{
"rainfall": {
"rainfall": 11.11
}
}
62. Ultraviolet Index (ultraviolet-index)
62.1. Capability Declaration Example:
[
{
"capability": "ultraviolet-index",
"permission": "0100",
"settings": {
"ultravioletIndexRange": {
"type": "numeric",
"permission": "01",
"min": 0, // Minimum value. **Type:** Number. Required. Must be less than `max`.
"max": 16 // Maximum value. **Type:** Number. Required. Must be greater than `min`.
}
}
}
]
62.2. Attributes (State):
{
"ultravioletIndex": 11.1 // Ultraviolet index. **Type:** Number. Required.
}
62.3. Protocol (Query Status & Control Instructions):
{
"ultraviolet-index": {
"ultravioletIndex": 11.1
}
}
63. Electrical Conductivity (electrical-conductivity)
63.1. Capability Declaration Example:
[
{
"capability": "electrical-conductivity",
"permission": "0100",
"settings": {
"electricalConductivityRange": {
"type": "numeric",
"permission": "01",
"min": 0, // Minimum value. **Type:** Number. Required. Must be less than `max`.
"max": 23 // Maximum value. **Type:** Number. Required. Must be greater than `min`.
}
}
}
]
63.2. Attributes (State):
{
"electricalConductivity": 11.11 // Electrical conductivity value. **Type:** Number. Required. **Unit:** dS/m.
}
63.3. Protocol (Query Status & Control Instructions):
{
"electrical-conductivity": {
"electricalConductivity": 11.11
}
}
64. Transmit Power (transmit-power)
64.1. Capability Declaration Example:
[
{
"capability": "transmit-power",
"permission": "1100"
}
]
64.2. Attributes (State):
{
"transmitPower": 9 // Device transmit power value. **Type:** Integer. Required. **Unit:** dBm.
}
64.3. Protocol (Query Status & Control Instructions):
{
"transmit-power": {
"transmitPower": 9
}
}
65. PM2.5 Detection (pm25)
65.1. Capability Declaration Example:
[
{
"capability": "pm25",
"permission": "0100"
}
]
65.2. Attributes (State):
{
"pm25": 10 // PM2.5 concentration in the air. **Type:** Number. Required. **Unit:** µg/m³.
}
65.3. Protocol (Query Status & Control Instructions):
{
"pm25": {
"pm25": 10
}
}
66. VOC Index (voc-index)
66.1. Capability Declaration Example:
{
"capability": "voc-index",
"permission": "0100"
}
66.2. Attributes (State):
{
"vocIndex": 10 // VOC index reflecting the level of harmful gas pollution. **Type:** Number. Required. **Unit:** µg/m³.
}
66.3. Protocol (Query Status & Control Instructions):
{
"voc-index": {
"vocIndex": 10
}
}
67. Natural Gas Detection (gas)
67.1. Capability Declaration Example:
{
"capability": "gas",
"permission": "0100"
}
67.2. Attributes (State):
{
"gas": true // Indicates whether natural gas leakage is detected. **Type:** Boolean. Required.
}
67.3. Protocol (Query Status & Control Instructions):
{
"gas": {
"gas": true
}
}
68. Irrigation Work Status (irrigation/work-status)
68.1. Capability Declaration Example:
[
{
"capability": "irrigation",
"permission": "0101",
"name": "work-status",
"settings": {
"volumeUnit": {
"type": "enum",
"permission": "01",
"values": ["L"],
"value": "L"
}
}
}
]
68.2. Attributes (State):
{
"realTimeVolume": 20, // Real-time irrigation volume. **Type:** Number. Optional. **Unit:** L.
"start": "2020-07-05T08:00:00Z", // Irrigation start time. **Type:** Date. Optional.
"end": "2020-07-05T09:00:00Z", // Irrigation end time. **Type:** Date. Optional.
"dailyVolume": 20 // Daily irrigation volume. **Type:** Number. Optional. **Unit:** L.
}
68.3. Protocol (Query Status & Control Instructions):
Work Status Reporting:
{
"irrigation": {
"work-status": {
"realTimeVolume": 20,
"start": "2020-07-05T08:00:00Z",
"end": "2020-07-05T09:00:00Z",
"dailyVolume": 20
}
}
}
Work Status Query:
{
"irrigation": {
"type": "oneDay", // Aggregation type. **Type:** String. Required. Options: "oneDay", "30Days", "180Days".
"timeRange": { // Time range for aggregation. **Type:** Object. Required.
"start": "2020-07-05T08:00:00Z", // Start time for irrigation data aggregation. **Type:** String. Required.
"end": "2020-07-05T09:00:00Z" // End time for irrigation data aggregation. **Type:** String. Optional. If omitted, current time is used.
}
}
}
Work Status Query Result:
{
"irrigation": {
"type": "oneDay", // Aggregation type. **Type:** String. Required.
"irrigationIntervals": [
{
"volume": 6500, // Irrigation volume. **Type:** Number. Required. **Unit:** L.
"duration": 30, // Irrigation duration. **Type:** Number. Required. **Unit:** minutes.
"start": "2020-07-05T08:00:00Z", // Start time. **Type:** String. Required.
"end": "2020-07-05T09:00:00Z" // End time. **Type:** String. Required.
},
{
"volume": 6500, // Irrigation volume. **Type:** Number. Required. **Unit:** L.
"duration": 30, // Irrigation duration. **Type:** Number. Required. **Unit:** minutes.
"start": "2020-07-05T08:00:00Z", // Start time. **Type:** String. Required.
"end": "2020-07-05T09:00:00Z" // End time. **Type:** String. Required.
}
]
}
}
69. Irrigation Work Mode (irrigation/work-mode)
69.1. Capability Declaration Example:
[
{
"capability": "irrigation",
"permission": "0100",
"name": "work-mode",
"settings": {
"supportedModes": {
"type": "enum",
"permission": "01",
"values": [
"MANUAL", // Manual mode
"TIMER", // Single-time scheduling
"CYCLE-TIMER", // Repeated scheduling
"VOLUME", // Single-time volume
"CYCLE-VOLUME" // Repeated volume
]
}
}
}
]
69.2. Attributes (State):
{
"workMode": "MANUAL" // Irrigation work mode. **Type:** String. Optional. Values should be from `settings.supportedModes`.
}
69.3. Protocol (Query Status & Control Instructions):
{
"irrigation": {
"work-mode": "MANUAL"
}
}
70. Automatic Irrigation Controller (irrigation/auto-controller)
70.1. Capability Declaration Example:
[
{
"capability": "irrigation",
"permission": "1100",
"name": "auto-controller",
"settings": {
"volumeRange": { // Volume range
"type": "enum",
"permission": "01",
"min": 0,
"max": 6500,
"unit": "L"
},
"timeRange": { // Time range
"type": "enum",
"permission": "01",
"min": 1,
"max": 86400,
"unit": "second"
},
"cycleCountRange": { // Cycle count range
"type": "enum",
"permission": "01",
"min": 1,
"max": 100,
"step": 1
}
}
}
]
70.2. Attributes (State):
Single-Time or Repeated Scheduling:
{
"type": "time", // Irrigation mode. **Type:** String. Required. Options: "volume", "time".
"action": {
"perDuration": 60, // Duration per irrigation cycle. **Type:** Number. Required.
"intervalDuration": 20, // Interval between irrigation cycles. **Type:** Number. Required.
"count": 3 // Number of irrigation cycles. **Type:** Number. Required.
}
}
Single-Time or Repeated Volume:
{
"type": "volume", // Irrigation mode. **Type:** String. Required. Options: "volume", "time".
"action": {
"perConsumedVolume": 60, // Volume consumed per irrigation cycle. **Type:** Number. Required.
"intervalDuration": 20, // Interval between irrigation cycles. **Type:** Number. Required.
"count": 3 // Number of irrigation cycles. **Type:** Number. Required.
}
}
70.3. Protocol (Query Status & Control Instructions):
Single-Time or Repeated Scheduling:
{
"irrigation": {
"auto-controller": {
"type": "time", // Irrigation mode. **Type:** String. Required.
"action": {
"perDuration": 60, // Duration per irrigation cycle. **Type:** Number. Required.
"intervalDuration": 20, // Interval between cycles. **Type:** Number. Required.
"count": 3 // Number of cycles. **Type:** Number. Required.
}
}
}
}
Single-Time or Repeated Volume:
{
"irrigation": {
"auto-controller": {
"type": "volume", // Irrigation mode. **Type:** String. Required.
"action": {
"perConsumedVolume": 60, // Volume consumed per cycle. **Type:** Number. Required.
"intervalDuration": 20, // Interval between cycles. **Type:** Number. Required.
"count": 3 // Number of cycles. **Type:** Number. Required.
}
}
}
}
Supported Preset Modes
Mode Names | Optional Values |
fanLevel (fan Level) | “low” “medium” “high” |
thermostatMode(Thermostat Mode) | “auto” “manual” |
airConditionerMode >= 1.11.0(airConditioner Mode) | “cool” “heat” “auto” “fan” “dry” |
fanMode >= 1.11.0(Fan Mode) | “normal” “sleep” “child” |
horizontalAngle >= 1.11.0(Horizontal Angle) | “30” “60” “90” “120” “180” “360” |
verticalAngle >= 1.11.0(Vertical Angle) | “30” “60” “90” “120” “180” “360” |
c. Tags Description
- The special key toggle is used to set the name of the toggle subcomponent.
{
"toggle": {
'1': 'Chanel1',
'2': 'Chanel2',
'3': 'Chanel3',
'4': 'Chanel4',
},
}
- The special key temperature_unit is used to set the temperature unit.
{
"temperature_unit":'c' // c-Celsius; f-Fahrenheit
}
d. Special Error Code and Description
Error Code | Description | iHost Version |
110000 | The sub-device/group corresponding to the id does not exist | ≥2.1.0 |
110001 | The gateway is in the state of discovering zigbee devices | ≥2.1.0 |
110002 | Devices in a group do not have a common capability | ≥2.1.0 |
110003 | Incorrect number of devices | ≥2.1.0 |
110004 | Incorrect number of groups | ≥2.1.0 |
110005 | Device Offline | ≥2.1.0 |
110006 | Failed to update device status | ≥2.1.0 |
110007 | Failed to update group status | ≥2.1.0 |
110008 | The maximum number of groups has been reached. Create up to 50 groups | ≥2.1.0 |
110009 | The IP address of the camera device is incorrect | ≥2.1.0 |
110010 | Camera Device Access Authorization Error | ≥2.1.0 |
110011 | Camera device stream address error | ≥2.1.0 |
110012 | Camera device video encoding is not supported | ≥2.1.0 |
110013 | Device already exists | ≥2.1.0 |
110014 | Camera does not support offline operation | ≥2.1.0 |
110015 | The account password is inconsistent with the account password in the RTSP stream address | ≥2.1.0 |
110016 | The gateway is in the state of discovering onvif cameras | ≥2.1.0 |
110017 | Exceeded the maximum number of cameras added | ≥2.1.0 |
110018 | The path of the ESP camera is wrong | ≥2.1.0 |
110019 | Failed to access the service address of the third-party device | ≥2.1.0 |
e. Search for Sub-device
Allow authorized users to enable or disable gateway search for sub-devices through this interface
- 💡Note: Only supports searching for Zigbee sub-devices now.
- 💡Note: Zigbee sub-devices will be added automatically after searching. Do not need to use the “Manually Add Sub-devices” interface
- URL:/open-api/v2/rest/devices/discovery
- Method: PUT
- Header:
- Content-Type: application/json
- Autorization: Bearer <token>
Request parameters:
Attribute | Type | Optional | Description |
enable | boolean | N | true (Start paring); false (Pause pairing) |
type | string | N | Searching Type: Zigbee |
Successful data response:empty Object {}
Conditions: The request parameters are legal, and the user identity verification is passed.
Status Code: 200 OK
Response Example:
{
"error": 0,
"data": {},
"message": "success"
}
f. Manually Add the Sub-device
Allow authorized users to add a single sub-device through this interface.
- Note: Only RTSP Camera and ESP32 Camera are supported now
- URL:/open-api/v2/rest/devices
- Method:POST
- Header:
- Content-Type: application/json
- Autorization: Bearer <token>
Request parameters:
Attribute | Type | Optional | Description |
name | string | N | Sub-device name |
display_category | string | N | Device Type. Only support camera。 |
capabilities | CapabilityObject[] | N | Capability list. When display_category = camera, capabilities only include camera-stream capabilities. |
protocal | string | N | Device protocol. RTSP; ESP32-CAM |
manufacturer | string | N | Manufacturer. |
model | string | N | Device model |
firmware_version | string | N | Device firmware version |
CapabilityObject
Attribute | Type | Optional | Description |
capability | string | N | Capability name. Only support “camera-stream” |
permission | string | N | Device permission. Only support read. |
configuration | CameraStreamConfigurationObject | Y | Camera stream configuration. |
SettingsObject
Attribute | Type | Optional | Description |
streamSetting | StreamSettingObject | N | Stream service configuration |
StreamSettingObject
Attribute | Type | Optional | Description |
type | string | N | Stream service configuration |
permission | string | N | Capability permission. Only supports “11” (modifiable). |
value | StreamSettingValueObject | N | Specific configuration values |
StreamSettingValueObject
Attribute | Type | Optional | Description |
stream_url | string | N | Stream URL Format Format: Example: Schema Options:
*Note: Some cameras may not require a username or password. In such cases, you can omit the |
Successful data response:
Attribute | Type | Optional | Description |
serial_number | string | N | Device unique serial number |
Conditions: The request parameters are legal, and the user identity verification is passed.
Status Code: 200 OK
Response Example:
{
"error": 0,
"data": {
"serial_number": "serial_number"
},
"message": "success"
}
Failure data response: empty Object
Condition:
- Camera stream address access error (format error, authorization failure, network exception, etc.)
- Device already exists
- If a single device fails to add, returns all devices fail to add.
Status Code: 200 OK
Error code:
● 110009 Camera IP address error
● 110010 Camera access authorization error
● 110011 Camera stream address error
● 110012 The Camera video encoding does not support
● 110013 Device already exists
Response Example:
{
"error": 110009,
"data": {},
"message": "camera ip access failed"
}
g. Get List of Sub-devices
Allow authorized users to obtain the list of gateway sub-device through this interface.
- URL:/open-api/v2/rest/devices
- Method: GET
- Header:
- Content-Type: application/json
- Autorization: Bearer <token>
Request Parameters: none
Successful data response:
Attribute | Type | Optional | Description |
device_list | ResponseDeviceObject[] | N | Device list |
ResponseDeviceObject
Attribute | Type | Optional | Description |
serial_number | string | N | Device unique serial number |
third_serial_number | string | “N” when a third-party device is connected, otherwise “Y” | Third-party device unique serial number |
service_address | string | “N” when a third-party device is connected, otherwise “Y” | Third-party service address |
name | string | N | Device name, if it is not renamed, will be displayed by the front end according to the default display rules. |
manufacturer | string | N | Manufacturer |
model | string | N | Device model |
firmware_version | string | N | Firmware version. Can be an empty string. |
hostname | string | Y | Device hostname |
mac_address | string | Y | Device mac address |
app_name | string | Y | The name of the application to which it belongs. If the app_name is filled in when obtaining the open interface certificate, then all subsequent devices connected through the certificate will be written into this field. |
display_category | string | N | Device category |
capabilities | CapabilityObject[] | N | Device capabilities |
protocol | string | “N” when a third-party device is connected, otherwise “Y” | Device protocol: zigbee, onvif, rtsp, esp32-cam |
state | object | Y | Device state object. For state examples of different capabilities, please check 【Support Device Capabilities】 |
tags | object | Y | JSON format key-value, custom device information. The function is as follows:– Used to store device channels– Used to store temperature units– Custom information for other third-party devices |
online | boolean | N | Online status: True for onlineFalse is offline |
subnet | boolean | Y | Whether it is in the same subnet as the gateway |
CapabilityObject
💡Note: Please check the Examples of Supported Device Capabilities for [Supported Device Capabilities].
Attribute | Type | Optional | Description |
capability | string | N | Ability name. For details, check [Supported Device Capabilities] |
permission | string | N | Capability permission. Possible values are “read” (readable), “write” (writable), “readWrite” (readable and writable). |
configuration | object | Y | Capability configuration information. Currently camera-stream is used, check [Supported Device Capabilities] |
name | string | Y | The name field in toggle. The sub-channel number used to identify multi-channel devices. For example, if name is 1, it means channel 1. |
Conditions: The request parameters are legal, and the user identity verification is passed.
Status Code: 200 OK
Response Example:
{
"error": 0,
"data":{
"device_list": [
{
"serial_number": "ABCDEFGHIJK",
"name": "My Device",
"manufacturer": "SONOFF",
"model": "BASICZBR3",
"firmware_version": "1.1.0",
"display_category": "switch",
"capabilities": [
{
"capability": "power",
"permission": "readWrite"
},
{
"capability": "rssi",
"permission": "read"
}
],
"protocal": "zigbee",
"state": {
"power": {
"powerState": "on"
}
},
"tags": {
"key": "value"
},
"online": true
}
],
}
"message": "success"
}
h. Update Specified Device Information or State
Allow authorized users to modify the basic information of sub-device and issue control commands through this interface.
- URL:/open-api/v2/rest/devices/{serial_number}
- Method:PUT
- Header:
- Content-Type: application/json
- Autorization: Bearer <token>
Request parameters:
Attribute | Type | Optional | Description |
name | string | Y | Device name |
tags | object | Y | JSON format key-value, custom device information. |
state | object | Y | Device state object. For state examples of different capabilities, please check [Support Device Capabilities] |
configuration | object | Y | Capability configuration information, currently only the camera_stream capability supports modification. |
Successful data response:
Conditions: The request parameters are legal, and the user identity verification is passed.
Status Code: 200 OK
Error Code:
- 110000 The sub-device/group corresponding to the id does not exist
Response Example:
{
"error": 0,
"data":{
"serial_number": "ABCDEFGHIJK",
"third_serial_number": "third_serial_number",
"name": "我的设备",
"manufacturer": "SONOFF",
"model": "BASICZBR3",
"firmware_version": "1.1.0",
"display_category": "switch",
"capabilities": [
{
"capability": "power",
"permission": "1100"
},
{
"capability": "rssi",
"permission": "0100"
}
],
"protocal": "zigbee",
"state": {
"power": {
"powerState": "on"
}
},
"tags": {
"key": "value"
},
"online": true
},
"message": "success"
}
i. Delete Sub-device
Allow authorized users to delete sub-devices through this interface.
- URL:/open-api/v2/rest/devices/{serial_number}
- Method:DELETE
- Header:
- Content-Type: application/json
- Autorization: Bearer <token>
Request Parameters:
Attribute | Type | Optional | Description |
name | string | Y | Device name. |
tags | object | Y | Key-value pairs in JSON format used to store device channel names and other information about sub-devices. |
state | object | Y | Change device status; for specific protocol details, refer to “Supported Device Capabilities.” |
capabilities | CapabilityObject [] | Y | Capability configuration information; all capabilities that support setting configurations can be modified. Note that permissions cannot be changed. |
Successful data response:
Conditions: The request parameters are legal, and the user identity verification is passed.
Status Code: 200 OK
Error Codes:
- 110000: The sub-device/group corresponding to the ID does not exist.
- 110006: Failed to update device status.
- 110019: Failed to access third-party device service address.
- 110024: Failed to update device configuration.
Response Example:
{
"error": 0,
"data": {},
"message": "success"
}
import axios from 'axios';
const serial_number = 'serial_number';
const access_token = 'access_token';
(async function main() {
// turn on device
await axios({
url: `http://<domain name or ip address>/open-api/v2/rest/devices/${serial_number}`,
method: 'PUT',
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${access_token}`
},
data: {
state: {
power: {
powerState: 'on'
}
}
}
});
})()
j. Delete Sub-Device
Allows authorized users to delete sub-devices through this interface.
- URL:
/open-api/v2/rest/devices/{serial_number}
- Method:
DELETE
- Header:
- Content-Type: application/json
- Authorization: Bearer <token>
Request parameters: None
Successful data response:
Conditions: The request parameters are legal, and the user identity verification is passed.
Status Code: 200 OK
Response Example:
{
"error": 0,
"data": {},
"message": "success"
}
k. Query Device Status
Allows authorized users to query device status through this interface.
- URL:
/open-api/v2/rest/devices/:serial_number/query-state/{capability}
- Method:POST
- Header:
- Content-Type: application/json
- Authorization: Bearer <token>
Request parameters:
Attribute | Type | Optional | Description |
query_state | object | N | Query device status; for specific protocol details, refer to “Supported Device Capabilities.” |
import axios from 'axios';
const serial_number = 'serial_number';
const access_token = 'access_token';
(async function main() {
// turn on device
await axios({
url: `http://<domain name or ip address>/open-api/v2/rest/devices/${serial_number}/query-state/power-consumption`,
method: 'PUT',
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${access_token}`
},
data: {
"query_state":{
"power-consumption":{
"type": "summarize", // Type of statistics, required
"timeRange": { // Time range for summarization, required when type="summarize"
"start": "2020-07-05T08:00:00Z", // Start time for power consumption statistics
"end": "2020-07-05T09:00:00Z" // End time for power consumption statistics; if omitted, defaults to current time
}
}
}
});
})()
Successful data response:
Conditions: The request parameters are legal, and the user identity verification is passed.
Status Code: 200 OK
Response Example:
{
"error": 0,
"data": {},
"message": "success"
}
3.4 Security Management
a. Get Security List
Allows authorized users to modify gateway settings through this interface.
- URL:
/open-api/v2/rest/security
- Method:
GET
- Header:
- Content-Type: application/json
- Authorization: Bearer <token>
Request parameters: None
Successful data response:
Attribute | Type | Optional | Description |
security_list | ResponseSecurityObject[] | N | 响应设备列表 |
ResponseDeviceObject
Attribute | Type | Optional | Description |
sid | int | N | 安防id |
name | string | N | 安防名称 |
enable | bool | N | 是否启用
|
Conditions: The request parameters are legal, and the user identity verification is passed.
Status Code: 200 OK
Response Example:
{
"error": 0,
"data": {
"security_list":[
{
"sid": 1,
"name": "Home Mode",
"enable": true
}
]
},
"message": "success"
}
b. Enable Specified Security Mode
Allows authorized users to enable a specified security mode through this interface.
- URL:
/open-api/v2/rest/security/{security_id}/enable
- Method:
PUT
- Header:
- Content-Type: application/json
- Authorization: Bearer <token>
Request parameters: None
Successful data response:empty Object {}
Conditions: The request parameters are legal, and the user identity verification is passed.
Status Code: 200 OK
Response Example:
{
"error": 0,
"data": {},
"message": "success"
}
c. Disable Specified Security Mode
Allows authorized users to disable a specified security mode through this interface.
- URL:
/open-api/v2/rest/security/{security_id}/disable
- Method:
PUT
- Header:
- Content-Type: application/json
- Authorization: Bearer <token>
Request parameters: None
Successful data response:empty Object {}
Conditions: The request parameters are legal, and the user identity verification is passed.
Status Code: 200 OK
Response Example:
{
"error": 0,
"data": {},
"message": "success"
}
d. One-Click Enable Security Setup
Allows authorized users to enable the one-click security setup mode through this interface.
- URL:
/open-api/v2/rest/security/enable
- Method:
PUT
- Header:
- Content-Type: application/json
- Authorization: Bearer <token>
Request parameters: None
Successful data response:empty Object {}
Conditions: The request parameters are legal, and the user identity verification is passed.
Status Code: 200 OK
Response Example:
{
"error": 0,
"data": {},
"message": "success"
}
e. Disable Security
Allows authorized users to disable security through this interface.
- URL:
/open-api/v2/rest/security/disable
- Method:
PUT
- Header:
- Content-Type: application/json
- Authorization: Bearer <token>
Request parameters: None
Successful data response:empty Object {}
Conditions: The request parameters are legal, and the user identity verification is passed.
Status Code: 200 OK
Response Example:
{
"error": 0,
"data": {},
"message": "success"
}
4. Third-party Device Access
4.1 Access Instruction
Device Access
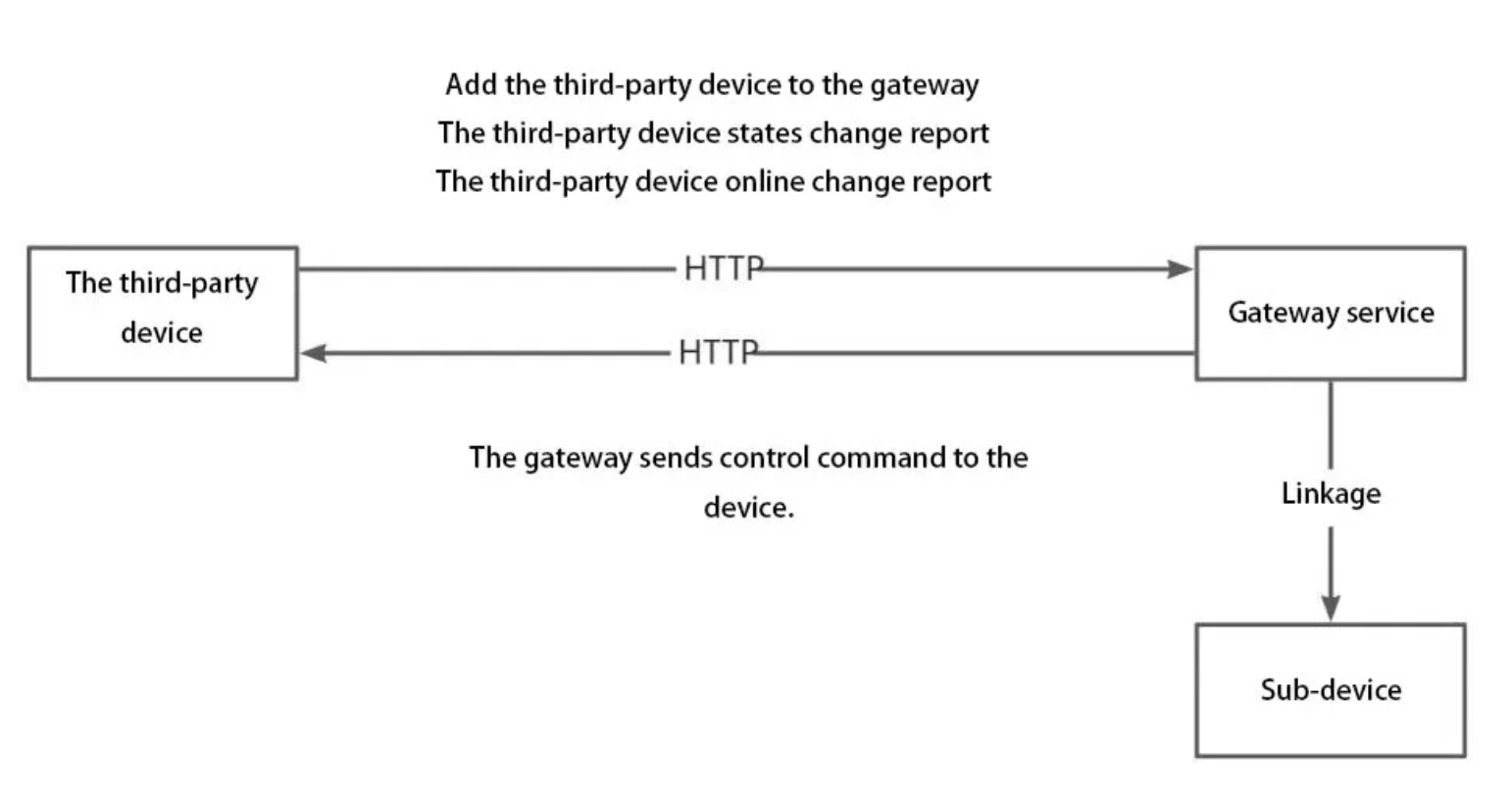
Third-party gateway Access
- Determine the classification of the device in the gateway. The detail please check the [Supported Device Type].
- Determine the capabilities that the device can access. The detail please check the [Supported Device Capabilities].
- Request the [Discovery Request] interface to add the device to the gateway.
-
- Attention: You need to provide the service address for receiving the instructions issued by the gateway, which is used to receive the device control instructions issued by the gateway.
- If the status of the third-party device changes, you need to call the [Device States Change Report] interface to send the latest status back to the gateway.
- After adding, the third-party device will appear in the Device List, with most of the functions of the gateway (other non-third-party devices). The common open interfaces related to the device can be used normally.
- Select the appropriate device category. The classification will affect the final display result of the UI after the device is connected to the gateway.
- Choose the right device capability. The capability list will determine the status of the device state protocol.
Program Process
a. Device Access
b. Third-party Gateway Access
4.2 Access Example
Switch, Plug
Sync third-party devices
// Request
URL:/open-api/v2/rest/thirdparty/event
Method:POST
Header:
Content-Type: application/json
Autorization: Bearer <token>
Body:
{
"event": {
"header": {
"name": "DiscoveryRequest",
"message_id": "Unique identifier, preferably a version 4 UUID",
"version": "2"
},
"payload": {
"endpoints": [
{
"third_serial_number": "third_serial_number_1",
"name": "my plug",
"display_category": "plug",
"capabilities": [
{
"capability": "power",
"permission": "1100"
}
],
"state": {
"power": {
"powerState": "on"
}
},
"tags": {
"key": "value"
},
"manufacturer": "manufacturer name",
"model": "model name",
"firmware_version": "firmware version",
"service_address": "http://192.168.31.14/webhook"
}
]
}
}
}
{
"header": {
"name": "Response",
"message_id": "Unique identifier, preferably a version 4 UUID",
"version": "1"
},
"payload": {
"endpoints": [
{
"third_serial_number": "third_serial_number",
"serial_number": "serial number"
}
]
}
}
Report device status
URL:/open-api/v2/rest/thirdparty/event
Method:POST
Header:
Content-Type: application/json
Autorization: Bearer <token>
Body:
{
"event": {
"header": {
"name": "DeviceStatesChangeReport",
"message_id": "Unique identifier, preferably a version 4 UUID",
"version": "1"
},
"endpoint": {
"serial_number": "serial_number"
},
"payload": {
"state": {
"power": {
"powerState": "on"
}
}
}
}
}
{
"header": {
"name": "Response",
"message_id": "Unique identifier, preferably a version 4 UUID",
"version": "1"
},
"payload": {}
}
Report offline/online
{
"event": {
"header": {
"name": "DeviceStatesChangeReport",
"message_id": "Unique identifier, preferably a version 4 UUID",
"version": "1"
},
"endpoint": {
"serial_number": "serial_number"
},
"payload": {
"online": true
}
}
}
{
"header": {
"name": "Response",
"message_id": "Unique identifier, preferably a version 4 UUID",
"version": "1"
},
"payload": {}
}
Receive the instructions about the gateway control device
URL:<service address>
Method:POST
Header:
Content-Type: application/json
B
{
"directive": {
"header": {
"name": "UpdateDeviceStates",
"message_id": "Unique identifier, preferably a version 4 UUID",
"version": "1"
},
"endpoint": {
"third_serial_number": "third_serial_number",
"serial_number": "serial_number"
},
"payload": {
"state": : {
"power": {
"powerState": "on"
}
}
}
}
}
{
"header": {
"name": "Response",
"message_id": "Unique identifier, preferably a version 4 UUID",
"version": "1"
},
"payload": {}
}
Query device
URL:/open-api/v2/rest/devices
Method:GET
Header:
Content-Type: application/json
Autorization: Bearer <token>
Body: 无
{
"error": 0,
"data": {
"device_list": [
{
"serial_number": "serial number",
"third_serial_number": "third_serial_number",
"name": "my plug",
"manufacturer": "manufacturer name",
"model": "model name",
"firmware_version": "firmware version",
"display_category": "plug",
"capabilities": [
{
"capability": "power",
"permission": "readWrite"
}
],
"state": {
"power": {
"powerState": "on"
}
},
"tags": {
"key": "value"
},
"online": true
}
]
},
"message": "success"
}
4.3 Web API
Third-Party Request Gateway Interface
Request Format
Allow authorized users to send event requests to the gateway through this interface.
- URL:/open-api/v2/rest/thirdparty/event
- Method:POST
- Header:
-
- Content-Type: application/json
- Autorization: Bearer <token>
Request parameters:
Attribute |
Type |
Optional |
Description |
event |
EventObject |
N |
Request event object structure information |
EventObject
Attribute |
Type |
Optional |
Description |
header |
HeaderObject |
N |
Request header structure information |
endpoint |
EndpointObject |
Y |
Request endpoint structure informationNote: This field is empty when sync a new device list. |
payload |
PayloadObject |
N |
Request payload structure information |
HeaderObject
Attribute |
Type |
Optional |
Description |
name |
string |
N |
Request name. optional parameter: DiscoveryRequest: Sync new devices DeviceStatesChangeReport: Device status update report DeviceOnlineChangeReport: Device online status report |
message_id |
string |
N |
Request message ID, UUID_V4 |
version |
string |
N |
Request protocol version number. Currently fixed at 1 |
EndpointObject
Attribute |
Type |
Optional |
Description |
serial_number |
string |
N |
Device unique serial number |
third_serial_number |
string |
N |
Third-party device unique serial number |
tags |
object |
Y |
JSON format key-value, custom device information. [Device Management Function] – [Tags Description] |
PayloadObject
According to the different header.name have different request structure.
Response Format
Status Code: 200 OK
Response parameters:
Attribute |
Type |
Optional |
Description |
header |
HeaderObject |
N |
Response header structure information |
payload |
PayloadObject |
N |
Response payload structure information |
HeaderObject
Attribute |
Type |
Optional |
Description |
name |
string |
N |
Response header name. optional parameters:Response: Successful response ErrorResponse: Error response |
message_id |
string |
N |
Response header message ID, UUID_V4. Pass in the request message header.message_id here*If the request input parameter lacks message_id, this field will be an empty string when responding. |
version |
string |
N |
– Request protocol version number. Currently fixed at 1. |
Successful response–PayloadObject :
Depending on the request type, the response structure is different. For details, please refer to the specific request instruction document.
Failure response–PayloadObject:
Attribute |
Type |
Optional |
Description |
type |
string |
N |
Error Types:
|
description |
string |
N |
Error description |
DiscoveryRequest Sync a new device list
- Note: After the device is synchronized to the gateway, it is online by default, that is, online=true. Subsequent online changes are completely dependent on synchronization with the third party through the DeviceOnlineChangeReport interface.
Request parameters:
EndpointObject:None
PayloadObject:
Attribute |
Type |
Optional |
Description |
endpoints |
EndpointObject[] |
N |
Device List |
EndpointObject:
Attribute |
Type |
Optional |
Description |
third_serial_number |
string |
N |
Third-party device unique serial number |
name |
string |
N |
Device name |
display_category |
string |
N |
Device Category. See the [Supported Device Type] for details. *Three-party devices don’t support adding cameras now. |
capabilities |
CapabilityObject[] |
N |
Capabilities list |
state |
object |
N |
Initial state information |
manufacturer |
string |
N |
Manufacturer |
model |
string |
N |
Device model |
tags |
object |
Y |
JSON format key-value, custom device information:Be used to store device channelsBe used to store temperature unitsOther third-party custom data |
firmware_version |
string |
N |
Firmware version |
service_address |
string |
N |
Service address. Such as http://192.168.31.14/service_address |
Example of Request :
{
"event": {
"header": {
"name": "DiscoveryRequest",
"message_id": "Unique identifier, preferably a version 4 UUID",
"version": "1"
},
"payload": {
"endpoints": [
{
"third_serial_number": "third_serial_number_1",
"name": "my plug",
"display_category": "plug",
"capabilities": [
{
"capability": "power",
"permission" "readWrite"
}
],
"state": {
"power": {
"powerState": "on"
}
},
"tags": {
"key": "value"
},
"manufacturer": "manufacturer name",
"model": "model name",
"firmware_version": "firmware version",
"service_address": "http://192.168.31.14/service_address"
}
]
}
}
}
Response parameters:
PayloadObject:
Attribute |
Type |
Optional |
Description |
endpoints |
EndpointObject[] |
N |
Device list |
EndpointObject:
Attribute |
Type |
Optional |
Description |
serial_number |
string |
N |
Device unique serial number |
third_serial_number |
string |
N |
Third-party device unique serial number |
Example of a correct response:
{
"header": {
"name": "Response",
"message_id": "Unique identifier, preferably a version 4 UUID",
"version": "1"
},
"payload": {
"endpoints": [
{
"serial_number": "serial number",
"third_serial_number": "third_serial_number"
}
]
}
}
Example of an error response:
{
"header": {
"name": "ErrorResponse",
"message_id": "Unique identifier, preferably a version 4 UUID",
"version": "1"
},
"payload": {
"type": "INVALID_PARAMETERS",
"description": "webhook cannot be empty"
}
}
DeviceStatesChangeReport Device status change report
- Note: Repeated status reports may falsely trigger associated scene.
Request parameters:
PayloadObject:
Attribute |
Type |
Optional |
Description |
state |
object |
N |
Devicce state, See [Supported device cabilities] for detail |
Example of Request :
{
"event": {
"header": {
"name": "DeviceStatesChangeReport",
"message_id": "Unique identifier, preferably a version 4 UUID",
"version": "2"
},
"endpoint": {
"serial_number": "serial_number",
"third_serial_number": "third_serial_number",
},
"payload": {
"state": {
"power": {
"powerState": "on"
}
}
}
}
}
Response parameters:
PayloadObject: Empty Object
Example of a successful response:
{
"header": {
"name": "Response",
"message_id": "Unique identifier, preferably a version 4 UUID",
"version": "1"
},
"payload": {}
}
DeviceOnlineChangeReport Device online status report
- Note: Repeated status reports may falsely trigger associated scene.
Request parameters:
PayloadObject:
Attribute |
Type |
Optional |
Description |
online |
boolean |
N |
Device online status true: Online false: Offline |
Example of Request :
{
"event": {
"header": {
"name": "DeviceOnlineChangeReport",
"message_id": "Unique identifier, preferably a version 4 UUID",
"version": "2"
},
"endpoint": {
"serial_number": "serial_number",
"third_serial_number": "third_serial_number"
},
"payload": {
"online": true
}
}
}
Response parameters:
PayloadObject: Empty Object
Example of a successful response:
{
"header": {
"name": "Response",
"message_id": "Unique identifier, preferably a version 4 UUID",
"version": "1"
},
"payload": {}
}
DeviceInformationUpdatedReport Device Information Updated Report
- Note: Updating may affect existing scenes or security functions.
Request parameters:
PayloadObject:
Attribute |
Type |
Optional |
Description |
capabilities |
CapabilityObject[] |
N |
Capabilities List. Details can be found in the supported device capabilities section. Note: This parameter will only update the For the specific structure definition of the |
tags |
object |
Y |
JSON format key-value, custom device information.
|
Example of Request :
{
"event": {
"header": {
"name": "DeviceInformationUpdatedReport",
"message_id": "Unique identifier, preferably a version 4 UUID",
"version": "2"
},
"endpoint": {
"serial_number": "serial_number",
"third_serial_number": "third_serial_number"
},
"payload": {
"capabilities": [
{
"capability": "detect",
"permission": "0110",
"settings":{
"detectInterval":{
"permission": "11",
"type": "numeric",
"value": 300,
},
"detectSensitivity":{
"permission": "11",
"type": "numeric",
"value": 1000,
}
}
}
]
}
}
}
esponse parameters:
PayloadObject: Empty Object
Example of a successful response:
{
"header": {
"name": "Response",
"message_id": "Unique identifier, preferably a version 4 UUID",
"version": "2"
},
"payload": {}
}
Gateway sends the instruction interface through the device service address
- Note:
- The three parties need to respond to the gateway’s request within 3s. Otherwise, the gateway will judge the command processing timeout.
- If the third-party service is offline, the gateway will set the device to an offline state, and the third-party service needs to report the device state (DeviceStatesChangeReport) or the online state (DeviceOnlineChangeReport) before returning to the online state.
Request format
Gateway sends instructions to the third-party through the device service address interface.
- URL:<service address>
- Method:POST
- Header:
-
- Content-Type: application/json
Request parameters:
Attribute |
Type |
Optional |
Description |
directive |
DirectiveObject |
N |
Directive object structure information |
DirectiveObject
Attribute |
Type |
Optional |
Description |
header |
HeaderObject |
N |
Request header structure information |
endpoint |
EndpointObject |
N |
Request endpoint structure information |
payload |
PayloadObject |
N |
Request payload structure information |
HeaderObject
Attribute |
Type |
Optional |
Description |
name |
string |
N |
Request name. Optional parameters:UpdateDeviceStates: Update device states |
message_id |
string |
N |
Request message ID, UUID_V4 |
version |
string |
N |
Request protocol version number. Currently fixed at 1. |
EndpointObject
Attribute |
Type |
Optional |
Description |
serial_number |
string |
N |
Device unique serial number |
third_serial_number |
string |
N |
Third-party device unique serial number |
tags |
object |
N |
JSON format key-value, custom device information. [Device Management Function] – [Tags Description] |
PayloadObject:
According to different header.name
, there is a specific request structure for each.
Example of Request :
{
"directive": {
"header": {
"name": "UpdateDeviceStates",
"message_id": "Unique identifier, preferably a version 4 UUID",
"version": "1"
},
"endpoint": {
"serial_number": "serial_number",
"third_serial_number": "third_serial_number",
"tags": {}
},
"payload": {
"state": {
"power": {
"powerState": "on"
}
}
}
}
}
Response format
HTTP Status Code: 200 OK
HTTP Response Attribute:
Attribute |
Type |
Optional |
Description |
event |
EventObject |
N |
Response event structure information |
EventObject
Attribute |
Type |
Optional |
Description |
header |
HeaderObject |
N |
Request header structure information |
payload |
PayloadObject |
N |
Request payload structure information |
HeaderObject
Attribute |
Type |
Optional |
Description |
name |
string |
N |
Response header name. Optional parameter: UpdateDeviceStatesResponse: Update device states response ErrorResponse: Error response |
message_id |
string |
N |
Response header message ID, UUID_V4. Pass in the request message header.message_id here |
version |
string |
N |
Request protocol version number. Currently fixed at 1. |
Successful response–PayloadObject:
Depending on the request type, the response structure is different. For details, please refer to the specific request instruction document.
Failure response–PayloadObject:
Attribute |
Type |
Optional |
Description |
type |
string |
N |
Error Types:
|
Conditions: The request parameters are legal.
Status Code: 200 OK
Response parameters:
{
"event": {
"header": {
"name": "UpdateDeviceStatesResponse",
"message_id": "Unique identifier, preferably a version 4 UUID",
"version": "1"
},
"payload": {}
}
}
{
"event": {
"header": {
"name": "ErrorResponse",
"message_id": "Unique identifier, preferably a version 4 UUID",
"version": "1"
},
"payload": {
"type": "ENDPOINT_UNREACHABLE"
}
}
}
UpdateDeviceStates
Request parameters:
PayloadObject:
Attribute |
Type |
Optional |
Description |
state |
object |
N |
Devicce state, See [Supported device cabilities] for detail. |
Example of Request :
{
"directive": {
"header": {
"name": "UpdateDeviceStates",
"message_id": "Unique identifier, preferably a version 4 UUID",
"version": "1"
},
"endpoint": {
"serial_number": "serial_number"
},
"payload": {
"state": : {
"power": {
"powerState": "on"
}
}
}
}
}
Response parameters:
PayloadObject:empty Object
Example of Successful Response
{
"header": {
"name": "Response",
"message_id": "Unique identifier, preferably a version 4 UUID",
"version": "1"
},
"payload": {}
}
QueryDeviceStates
Request parameters:
PayloadObject:
Attribute |
Type |
Optional |
Description |
state |
object |
N |
Devicce state, See [Supported device cabilities] for detail. |
Example of Request :
{
"directive": {
"header": {
"name": "QueryDeviceStates",
"message_id": "Unique identifier, preferably a version 4 UUID",
"version": "2"
},
"endpoint": {
"serial_number": "serial_number",
"third_serial_number": "third_serial_number"
},
"payload": {
"state": {
"power-consumption": {
"timeRange": {
"start": "2020-07-05T08:00:00Z", // Start time for power consumption statistics, required.
"end": "2020-07-05T09:00:00Z" // End time for power consumption statistics, required.
}
}
}
}
}
}
Response parameters:
PayloadObject:
Attribute |
Type |
Optional |
Description |
state |
object |
N |
Devicce state, See [Supported device cabilities] for detail. |
Response example:
{
"event": {
"header": {
"name": "Response",
"message_id": "Unique identifier, preferably a version 4 UUID",
"version": "2"
},
"payload": {
"state": {
"power-consumption": {
"electricityIntervals": [ // Divided into multiple records based on configuration.resolution
{
"usage": 26.5, // Power consumption value, required. Type: number.
"start": "2020-07-05T08:00:00Z", // Start time, required. Type: date.
"end": "2020-07-05T09:00:00Z" // End time, required. Type: date. If the interval between end and start is less than resolution, all reported records are considered invalid.
},
{
"usage": 26.5, // Power consumption value, required. Type: number.
"start": "2020-07-05T09:00:00Z", // Start time, required. Type: date.
"end": "2020-07-05T10:00:00Z" // End time, required. Type: date. If the interval between end and start is less than resolution, all reported records are considered invalid.
}
]
}
}
}
}
}
ConfigureDeviceCapabilities
Request parameters:
PayloadObject:
Attribute |
Type |
Optional |
Description |
capabilities |
CapabilityObject[] |
N |
能力列表。详情可看支持的设备能力部分。注意,permission字段不可更改,传入同步时相同的值即可。 |
Example of Request :
{
"directive": {
"header": {
"name": "ConfigureDeviceCapabilities",
"message_id": "Unique identifier, preferably a version 4 UUID",
"version": "2"
},
"endpoint": {
"serial_number": "serial_number"
},
"payload": {
"capabilities": [
{
"capability": "thermostat-mode-detect",
"permission": "0110",
"name": "temperature", // Type of temperature control detection, required. Optional values: humidity (humidity detection), temperature (temperature detection)
"settings": {
"setpointRange": {
"permission": "11",
"type": "object",
"value": {
"supported": [ // Supported detection settings, required.
{
"name": "lowerSetpoint", // Minimum value the detection should maintain. Either lowerSetpoint or upperSetpoint must be provided.
"value": { // Detection range, optional. Fill in if there are preset conditions.
"value": 68.0, // Temperature or humidity value, required.
"scale": "f" // Temperature unit, required if name=temperature. Options: c (Celsius), f (Fahrenheit)
}
},
{
"name": "upperSetpoint", // Maximum value the detection should maintain. Either lowerSetpoint or upperSetpoint must be provided.
"value": { // Detection range, optional. Fill in if there are preset conditions.
"value": 68.0, // Temperature or humidity value, required.
"scale": "f" // Temperature unit, required if name=temperature. Options: c (Celsius), f (Fahrenheit)
}
}
]
}
},
"supportedModes": {
"type": "enum",
"permission": "01",
"values": [
"COMFORT",
"COLD",
"HOT"
]
}
}
}
]
}
}
}
Response parameters:
PayloadObject:empty Object
Example of Successful Response
{
"event": {
"header": {
"name": "Response",
"message_id": "Unique identifier, preferably a version 4 UUID",
"version": "2"
},
"payload": {}
}
}
5. Server-sent events
MDN EventSource interface description:https://developer.mozilla.org/en-US/docs/Web/API/Server-sent_events
5.1 Instruction
The gateway supports pushing messages to the client using SSE (Server-sent events). The client can monitor SSE messages after obtaining the access credentials and can parse the content of the push messages according to the development interface event notification protocol after receiving the messages pushed by the gateway. It should be noted that the gateway currently uses the HTTP1.1 protocol, so SSE on the browser side there will be a maximum of no more than 6 connections limit (Specific instructions can be found in the MDN EventSource interface description.)
5.2 Common Format
- URL:/open-api/v2/sse/bridge
- Method:
GET
Request parameters:
Name |
Type |
Optional |
Description |
access_token |
string |
N |
Access Token |
Note:
When requesting an SSE connection, the gateway will check the access_token, and it will return an authentication failure error if it is invalid. { “error”: 401, “data”: {}, “message”: “invalid access_token”}
<Module Name>#<Version>#<Message Type>
For example:
Module Name: device
Version: 1,v2,v3
Message Type: addDevice
Example:
const evtSource = new EventSource("http://<domain name or ip address>/open-api/v2/sse/bridge?access_token=xxxxxx");
evtSource.addEventListener('device#v2#addDevice',function (event) {
try {
const data = JSON.parse(event.data);
console.log('data', data);
} catch (error) {
console.error(`parse error`,error);
}
}
5.3 Device Module
a. Add Device Event
Module Name:device
Version:v2
Message Type:addDevice
event.data parameters:
Name |
Type |
Optional |
Description |
payload |
ResponseDeviceObjectObject – Interface the same with the Get Device List |
N |
device information |
Example:
// event.data
{
"payload": {
"serial_number": "ABCDEFGHIJK",
"third_serial_number": "third_serial_number",
"name": "Mydevice",
"manufacturer": "SONOFF",
"model": "BASICZBR3",
"firmware_version": "1.1.0",
"display_category": "switch",
"capabilities": [
{
"capability": "power",
"permission": "1100"
},
{
"capability": "rssi",
"permission": "0100"
}
],
"protocal": "zigbee",
"state": {
"power": {
"powerState": "on"
}
},
"tags": {
"key": "value"
},
"online": true
}
}
b. Update Device State Event
Module Name:device
Version:v2
Message Type:updateDeviceState
event.data parameters:
Name |
Type |
Optional |
Description |
endpoint |
EndpointObject |
N |
Device Information |
payload |
object。 Structure the same as the device state |
N |
Device Status Data |
EndpointObject:
Parameter |
Type |
Optional |
Description |
serial_number |
string |
N |
Device unique Serial Number |
third_serial_number |
string |
Y |
The unique serial number of the third-party device. For devices connected through open interfaces, this field is required. |
Example:
// event.data
{
"endpoint": {
"serial_number": "serial_number",
"third_serial_number": "third_serial_number"
},
"payload": {
"power": {
"powerState": "on"
},
"brightness": {
"brightness": 100
}
}
}
c. Update Device Info Event
Module Name:device
Version:v2
Message Type:updateDeviceInfo
event.data parameters:
Name |
Type |
Optional |
Description |
endpoint |
EndpointObject |
N |
Device Information |
payload |
DeviceChangeObject |
N |
Device Change Data |
EndpointObject:
Attribute |
Type |
Optional |
Description |
serial_number |
string |
N |
Device unique Serial Number |
third_serial_number |
string |
Y |
The unique serial number of the third-party device. For devices connected through open interfaces, this field is required. |
DeviceChangeObject
Name |
Type |
Optional |
Description |
name |
string |
N |
Device Name |
capabilities |
CapabilityObject [] |
Y |
Device capabilities list. |
tags |
object |
Y |
tags
|
Example:
// event.data
{
"endpoint": {
"serial_number": "serial_number",
"third_serial_number": "third_serial_number"
},
"payload": {
"name": "device name",
"capabilities": [
{
"capability": "thermostat-mode-detect",
"permission": "0110",
"name": "temperature", // Type of temperature control detection, required. Optional values: humidity (humidity detection), temperature (temperature detection)
"settings": {
"setpointRange": {
"permission": "11",
"type": "object",
"value": {
"supported": [ // Supported detection settings, required.
{
"name": "lowerSetpoint", // Minimum value the detection should maintain. Either lowerSetpoint or upperSetpoint must be provided.
"value": { // Detection range, optional. Fill in if there are preset conditions.
"value": 68.0, // Temperature or humidity value, required.
"scale": "f" // Temperature unit, required if name=temperature. Options: c (Celsius), f (Fahrenheit)
}
},
{
"name": "upperSetpoint", // Maximum value the detection should maintain. Either lowerSetpoint or upperSetpoint must be provided.
"value": { // Detection range, optional. Fill in if there are preset conditions.
"value": 68.0, // Temperature or humidity value, required.
"scale": "f" // Temperature unit, required if name=temperature. Options: c (Celsius), f (Fahrenheit)
}
}
]
}
},
"supportedModes": {
"type": "enum",
"permission": "01",
"values": [
"COMFORT",
"COLD",
"HOT"
]
}
}
}
]
}
}
d. Delete Device Event
Module Name:device
Version:v2
Message Type:deleteDevice
event.data parameters:
Name |
Type |
Optional |
Description |
endpoint |
EndpointObject |
N |
Device Information |
EndpointObject:
Attribute |
Type |
Optional |
Description |
serial_number |
string |
N |
Device unique Serial Number |
third_serial_number |
string |
Y |
The unique serial number of the third-party device. For devices connected through open interfaces, this field is required. |
Example:
// event.data
{
"endpoint": {
"serial_number": "serial_number",
"third_serial_number": "third_serial_number"
}
}
e. Update Device Online Event
Module Name:device
Version:v2
Message Type:updateDeviceOnline
event.data parameters:
Name |
Type |
Optional |
Description |
endpoint |
EndpointObject |
N |
Device Information |
payload |
DeviceChangeObject |
N |
Device Change Data |
EndpointObject:
Attribute |
Type |
Optional |
Description |
serial_number |
string |
N |
Device unique Serial Number |
third_serial_number |
string |
Y |
The unique serial number of the third-party device. For devices connected through open interfaces, this field is required. |
DeviceChangeObject
Name |
Type |
Optional |
Description |
online |
boolean |
N |
Device Online Status |
Example:
// event.data
{
"endpoint": {
"serial_number": "serial_number",
"third_serial_number": "third_serial_number"
},
"payload": {
"online": false
}
}
5.4 Gateway Module
a. Security State Update Event
Module Name:device
Version:v2
Message Type:updateDeviceOnline
event.data parameters:
Attribute |
Type |
Optional |
Description |
payload |
SecurityStateObject |
N |
Device Information |
SecurityStateObject
Attribute |
Type |
Optional |
Description |
alarm_state |
string |
N |
|
Example:
// event.data
{
"payload": {
"alarm_state": "alarming"
}
}
5.5 Security Module
a. Arm State Update Event
Module Name:device
Version:v2
Message Type:updateDeviceOnline
event.data parameters:
Attribute |
Type |
Optional |
Description |
payload |
ArmStateObject |
N |
rm and disarm information |
ArmStateObject:
Attribute |
Type |
Optional |
Description |
arm_state |
string |
N |
|
detail |
DetailObject |
N |
Arm/disarm details |
DetailObject:
Attribute |
Type |
Optional |
Description |
sid |
int |
N |
安防模式id |
name |
string |
N |
安防名称 |
Example
// event.data
{
"payload": {
"arm_state": "arming",
"detail": {
"sid": 1,
"name": "Home Mode"
}
}
}
6.1 Instruction
Key Role
- TTS Service Provider: The TTS service engine service provider is responsible for registering the TTS engine on the gateway and providing TTS services
- Gateway Server:iHost
- Gateway Open API Client
6.1.1 Registering TTS Engine Service
- 【TTS Service Provider】Call the interface to register the TTS engine on the gateway.
- 【Gateway Server】After successful registration, the gateway will store the basic information of the TTS engine (including the service address server_address, and subsequent communication between the gateway and the TTS Service Provider will be carried out through the server_address address), and allocate the TTS engine service ID within the gateway.
6.1.2 Get the List of Synthesized Audio
- 【Gateway Open API Client】Call the interface to obtain the list of registered TTS engine service. You can obtain the current list of registered TTS engines (including the ID of the TTS engine allocated by the gateway).
- 【Gateway Open API Client】Call the interface to obtain the list of a specified TTS engine audio. The gateway will issue a synchronous audio list instruction to the specified TTS Service Provider and return the result.
6.1.3 Speech Synthesis by Specifying a Speech Engine
- 【Gateway Open API Client】Call the interface to obtain the list of registered TTS engine service. You can obtain the current list of registered TTS engines (including the ID of the TTS engine allocated by the gateway).
- 【Gateway Open API Client】Call the interface to obtain the list of a specified TTS engine audio. The gateway will issue a synchronous audio list instruction to the specified TTS Service Provider and return the result.
6.1.4 Synthesize Audio and Play TTS Speech.
- 【Gateway Open API Client】Call the interface to obtain the list of registered TTS engine service. You can obtain the current list of registered TTS engines (including the ID of the TTS engine allocated by the gateway).
- 【Gateway Open API Client】Call the interface to obtain the list of a specified TTS engine audio. The gateway will issue a synchronous audio list instruction to the specified TTS Service Provider and return the result. (including the TTS audio file address)
- 【Gateway Open API Client】Record the TTS audio file address from the result returned in the previous step, call the play audio file interface, and play it through the gateway.
6.2 TTS Engine Module
6.2.1 Gateway Open Capability
a. Get the list of registered TTS engine services
- URL:
/open-api/v2/rest/tts/engines
- Method:
GET
- Header:
-
- Content-Type: application/json
- Authorization: Bearer <token>
Request Parameters: none
Correct data response:
Attribute |
Type |
Optional |
Description |
engines |
EngineObject [] |
N |
List of registered TTS engines |
EngineObject Structure
Attribute |
Type |
Optional |
Description |
id |
string |
N |
Engine ID assigned by gateway |
name |
string |
N |
Name of TS engine service |
Conditions: The request parameters are legal, and the user identity verification is passed.
Status Code: 200 OK
Response Example::
{
"error": 0,
"data": {
"engines": [
{
"id": "engine id",
"name": "engine name"
}
]
},
"message": "success"
}
b. Get list of specified TTS engine audio
- URL:
/open-api/v2/rest/tts/engine/{id}/audio-list
- Method:
GET
- Header:
-
- Content-Type: application/json
- Authorization: Bearer <token>
Request Parameters: none
Correct data response:
Attribute |
Type |
Optional |
Description |
audio_list |
AudioObject [] |
N |
Audio list |
AudioObject Structure
Attribute |
Type |
Optional |
Description |
url |
string |
N |
Audio file URL, for example: https://dl.espressif.cn/dl/audio/gs-16b-2c-44100hz.mp3 |
label |
string |
Y |
Audio file label |
Conditions: The request parameters are legal, and the user identity verification is passed.
Status Code: 200 OK
Error Code:
- 190000 The engine is running abnormally
Response Example::
{
"error": 0,
"data": {
"audio_list": [
{
"url": "tts audio address", // for example: https://dl.espressif.cn/dl/audio/gs-16b-2c-44100hz.mp3
"label": "tts audio label"
}
]
},
"message": "success"
}
c .Perform speech synthesis using the specified TTS engine
- URL:
/open-api/v2/rest/tts/engine/{id}/synthesize
- Method:
POST
- Header:
-
- Content-Type: application/json
- Authorization: Bearer <token>
Request Parameters:
Attribute |
Type |
Optional |
Description |
text |
string |
N |
Text used to synthesize the audio |
label |
string |
Y |
Audio file label |
language |
string |
Y |
Transparent field. Optional language code for the synthesis speech request. The specific list of supported language codes is provided by the TTS speech engine service provider. Note that the TTS engine service needs to support default language for speech synthesis, which means that if the language is not passed, the default language of the engine will be used for synthesis. |
options |
object |
Y |
Transparent field. It is used to pass the configuration parameters required for synthesis to the TTS speech engine service. |
Correct data response:
Attribute |
Type |
Optional |
Description |
audio |
AudioObject |
N |
Audio list |
AudioObject Structure
Attribute |
Type |
Optional |
Description |
url |
string |
N |
Audio file URL, for example: |
label |
string |
Y |
Audio file label |
Conditions: The request parameters are legal, and the user identity verification is passed.
Status Code: 200 OK
Error Code:
- 190000 The engine is running abnormally
Response Example::
{
"error": 0,
"data": {
"audio": {
"url": "tts audio address" // for example, https://dl.espressif.cn/dl/audio/gs-16b-2c-44100hz.mp3
"label": "tts audio label"
}
},
"message": "success"
}
6.2.2 Communication Between Gateway and TTS Service
a. Registering TTS engine service
Send request to gateway by TTS Service Provider
- URL:
/open-api/v2/rest/thirdparty/event
- Method:
POST
- Header:
-
- Content-Type: application/json
- Authorization: Bearer <token>
Request Parameters:
Attribute |
Type |
Optional |
Description |
event |
EventObject |
N |
Request Event Object structure Information |
EventObject
Attribute |
Type |
Optional |
Description |
header |
HeaderObject |
N |
Request header structure information |
payload |
PayloadObject |
N |
Request payload structure information |
HeaderObject
Attribute |
Type |
Optional |
Description |
name |
string |
N |
Request name. Optional parameter.
|
message_id |
string |
N |
Request message ID, UUID_V4 |
version |
string |
N |
Request protocol version number. Currently fixed at 1 |
PayloadObject
Attribute |
Type |
Optional |
Description |
service_address |
string |
N |
Service Address. For example, http://<domain name or ip address>/<path> |
name |
string |
N |
Service name |
Request Example:
{
"event": {
"header": {
"name": "RegisterTTSEngine",
"message_id": "Unique identifier, preferably a version 4 UUID",
"version": "1"
},
"payload": {
"service_address": "service_address",
"name": "tts service name"
}
}
}
Correct response parameters:
Attribute |
Type |
Optional |
Description |
header |
HeaderObject |
N |
Request header structure information |
payload |
PayloadObject |
N |
Request payload structure information |
HeaderObject
Attribute |
Type |
Optional |
Description |
name |
string |
N |
Response header name. Optional parameter:
|
message_id |
string |
N |
Response header message ID, UUID_V4. Incoming request message here: header.message_id |
version |
string |
N |
Request protocol version number. Currently fixed at 1 |
PayloadObject
Attribute |
Type |
Optional |
Description |
engine_id |
string |
N |
Engine ID assigned by gateway |
Conditions: The request parameters are legal, and the user identity verification is passed.
Status Code: 200 OK
Response Example::
Correct Response Example::
{
"header": {
"name": "Response",
"message_id": "Unique identifier, preferably a version 4 UUID",
"version": "1"
},
"payload": {
"engine_id": "engine id"
}
}
Abnormal response parameters:
Attribute |
Type |
Optional |
Description |
type |
string |
N |
Error Type
|
description |
string |
N |
Error description |
Error Response Example::
{
"header": {
"name": "ErrorResponse",
"message_id": "Unique identifier, preferably a version 4 UUID",
"version": "1"
},
"payload": {
"type": "INVALID_PARAMETERS",
"description": "service_address cannot be empty"
}
}
b. Synchronize audio list command
Send command to the TTS Service Provider by gateway.
- URL:
<service address>
- Method:
POST
- Header:
-
- Content-Type: application/json
Request Parameters:
Attribute |
Type |
Optional |
Description |
directive |
DirectiveObject |
N |
Instruction object structure information |
DirectiveObject
Attribute |
Type |
Optional |
Description |
header |
HeaderObject |
N |
Request header structure information |
payload |
PayloadObject |
N |
Request payload structure information |
HeaderObject
Attribute |
Type |
Optional |
Description |
name |
string |
N |
Request name. Optional parameter:
|
message_id |
string |
N |
Request message ID, UUID_V4 |
version |
string |
N |
Request protocol version number. Currently fixed at 1 |
Request Example:
{
"directive": {
"header": {
"name": "SyncTTSAudioList",
"message_id": "Unique identifier, preferably a version 4 UUID",
"version": "1"
},
"payload": {}
}
}
Correct response parameters:
Attribute |
Type |
Optional |
Description |
header |
HeaderObject |
N |
Request header structure information |
payload |
PayloadObject |
N |
Request payload structure information |
HeaderObject
Attribute |
Type |
Optional |
Description |
name |
string |
N |
Response header name. Optional parameter:
|
message_id |
string |
N |
Response header message ID, UUID_V4. Incoming request message here: header.message_id |
version |
string |
N |
Request protocol version number. Currently fixed at 1 |
PayloadObject:
Attribute |
Type |
Optional |
Description |
audio_list |
AudioObject [] |
N |
TTS Audio list |
AudioObject Structure
Attribute |
Type |
Optional |
Description |
url |
string |
N |
Audio file URL, for example: |
label |
string |
Y |
Audio file label |
Conditions: The request parameters are legal, and the user identity verification is passed.
Status Code: 200 OK
Response Example::
Correct Response Example::
{
"header": {
"name": "Response",
"message_id": "Unique identifier, preferably a version 4 UUID",
"version": "1"
},
"payload": {
"audio_list": [
{
"url": "tts audio url",
"label": "tts audio label"
}
]
}
}
Abnormal response parameters:
Attribute |
Type |
Optional |
Description |
type |
string |
N |
Error Type
|
description |
string |
N |
Error description |
Error Response Example::
{
"header": {
"name": "ErrorResponse",
"message_id": "Unique identifier, preferably a version 4 UUID",
"version": "1"
},
"payload": {
"type": "INVALID_PARAMETERS",
"description": "service_address cannot be empty"
}
}
c. Speech synthesis command
Send command to the TTS Service Provider by gateway.
- URL:
<service address>
- Method:
POST
- Header:
-
- Content-Type: application/json
Request Parameters:
Attribute |
Type |
Optional |
Description |
directive |
DirectiveObject |
N |
Instruction object structure information |
DirectiveObject
Attribute |
Type |
Optional |
Description |
header |
HeaderObject |
N |
Request header structure information |
payload |
PayloadObject |
N |
Request payload structure information |
HeaderObject
Attribute |
Type |
Optional |
Description |
name |
string |
N |
Request name. Optional parameter:
|
message_id |
string |
N |
Request message ID: UUID_V4 |
version |
string |
N |
Request protocol version number. Currently fixed at 1 |
PayloadObject
Attribute |
Type |
Optional |
Description |
text |
string |
N |
Text used to synthesize the audio |
label |
string |
Y |
Audio file label |
language |
string |
Y |
Transparent field. Optional language code for the synthesis speech request. The specific list of supported language codes is provided by the TTS speech engine service provider. Note that the TTS engine service needs to support default language for speech synthesis, which means that if the language is not passed, the default language of the engine will be used for synthesis. |
options |
object |
Y |
Transparent field. It is used to pass the configuration parameters required for synthesis to the TTS speech engine service. |
Request Example:
{
"directive": {
"header": {
"name": "SynthesizeSpeech",
"message_id": "Unique identifier, preferably a version 4 UUID",
"version": "1"
},
"payload": {
"text": "Input text to synthesize.",
"label": "tts audio label"
}
}
}
Correct response parameters:
Attribute |
Type |
Optional |
Description |
header |
HeaderObject |
N |
Request header structure information |
payload |
PayloadObject |
N |
Request payload structure information |
HeaderObject
Attribute |
Type |
Optional |
Description |
name |
string |
N |
Response header name. Optional parameter:
|
message_id |
string |
N |
Response header message ID, UUID_V4. Incoming request message here: header.message_id |
version |
string |
N |
Request protocol version number. Currently fixed at 1 |
PayloadObject
Attribute |
Type |
Optional |
Description |
audio |
AudioObject |
N |
TTS Audio |
AudioObject Structure
Attribute |
Type |
Optional |
Description |
url |
string |
N |
Audio file URL, for example: |
label |
string |
Y |
Audio file label |
Conditions: The request parameters are legal, and the user identity verification is passed.
Status Code: 200 OK
Response Example::
Correct Response Example::
{
"header": {
"name": "Response",
"message_id": "Unique identifier, preferably a version 4 UUID",
"version": "1"
},
"payload": {
"audio": {
"url": "tts audio url",
"label": "tts audio label"
}
}
}
Abnormal response parameters:
Attribute |
Type |
Optional |
Description |
type |
string |
N |
Error Type
|
description |
string |
N |
Error description |
Error Response Example::
{
"header": {
"name": "ErrorResponse",
"message_id": "Unique identifier, preferably a version 4 UUID",
"version": "1"
},
"payload": {
"type": "INVALID_PARAMETERS",
"description": "service_address cannot be empty"
}
}
7. Multimedia Module
7.1 Play Audio File
- URL:
/open-api/v2/rest/media/audio-player
- Method:
POST
- Header:
-
- Content-Type: application/json
- Authorization: Bearer <token>
Request Parameters:
Attribute |
Type |
Optional |
Description |
audio_url |
string |
N |
Audio URL address |
Correct data response:
Conditions: The request parameters are legal, and the user identity verification is passed.
Status Code: 200 OK
Response Example::
{
"error": 0,
"data": {},
"message": "success"
}
8. Custom UI Card
Custom UI cards allow you to display any content you want within the card. This content can be a webpage, an image, or any service with a UI. You just need to provide the URL of the content you wish to display.
The UI card will automatically adapt its width and height, and the content will be rendered using an iFrame.
8.1 Instruction
Key Role
- UI Service Provider: The provider responsible for creating custom UI cards on the gateway.
- Gateway Server: The gateway server (iHost).
- Gateway Open API Client: The Open API client for the gateway.
8.1.1 Creating a Custom UI Card
- [UI Service Provider]: Calls the API to create a custom UI card on the gateway.
- [Gateway Server]: Upon successful registration, the gateway stores the basic information of the UI card (including size configuration and card resource URL) and assigns an internal UI card ID within the gateway.
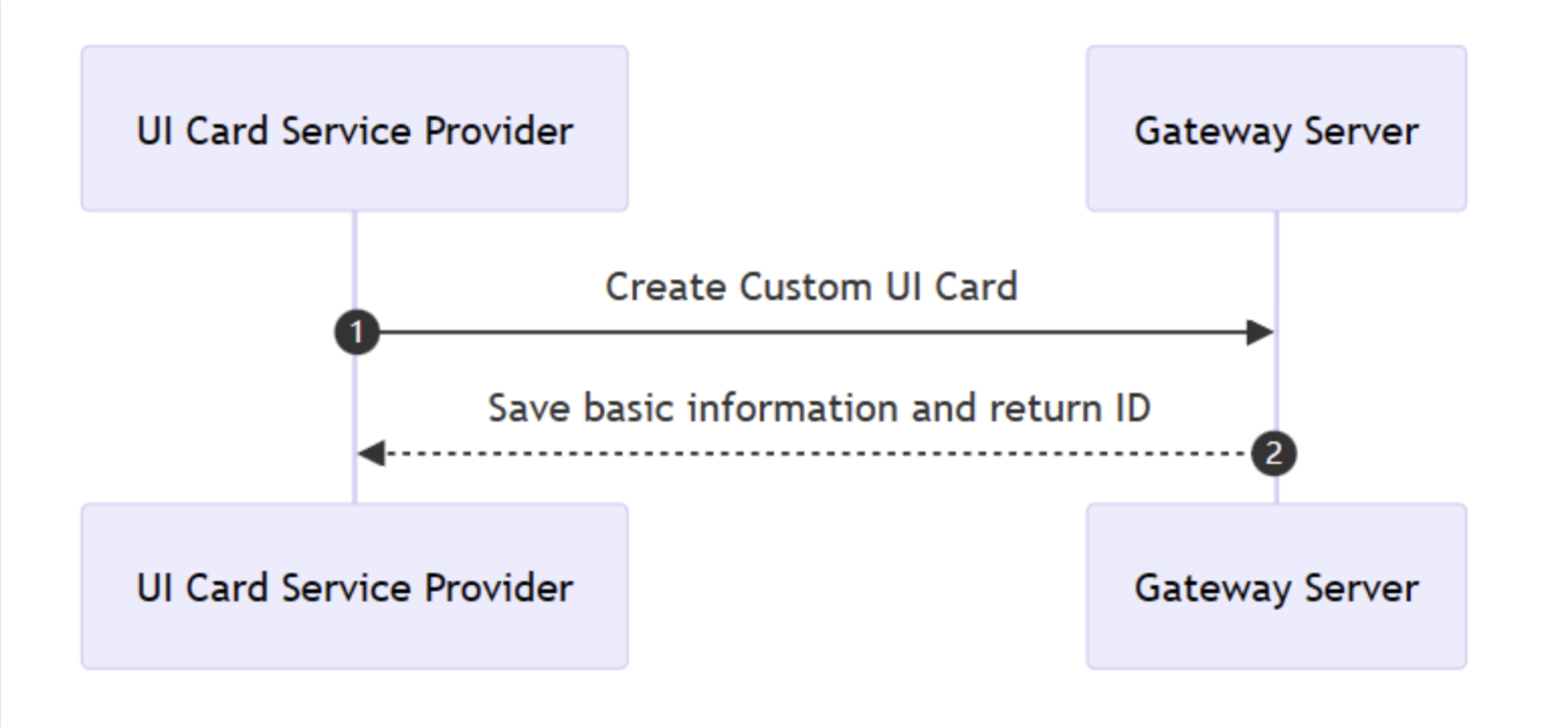
8.1.2 Retrieving the UI Card List
- [UI Service Provider]: Calls the API to retrieve the list of UI cards.
- [Gateway Server]: Returns the list of UI cards stored on the gateway, including custom UI cards not created by the caller.
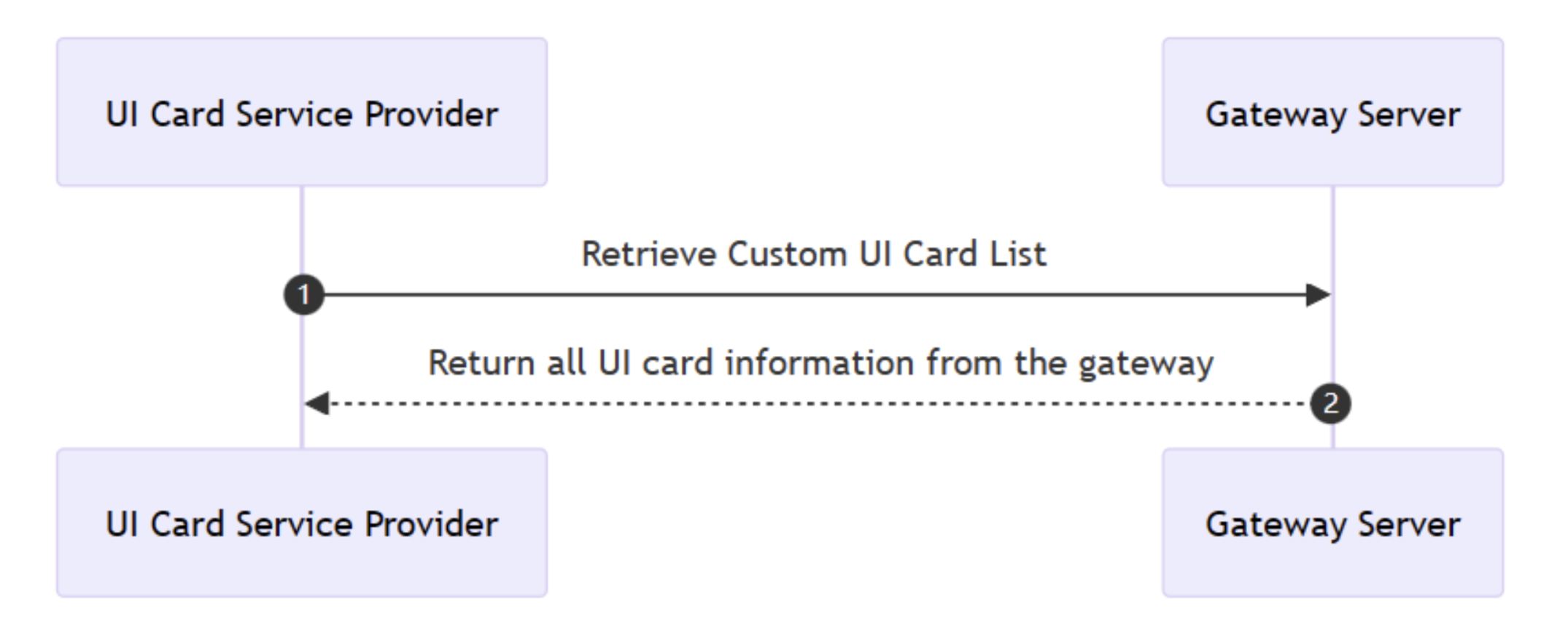
8.1.3 Modifying the Configuration of a Specified UI Card
- [UI Service Provider]: Calls the API to modify the configuration of a specified UI card, such as the size configuration and resource URL.
- [Gateway Server]: Upon successful modification, the gateway stores the updated UI card information, including the new size configuration and resource URL.
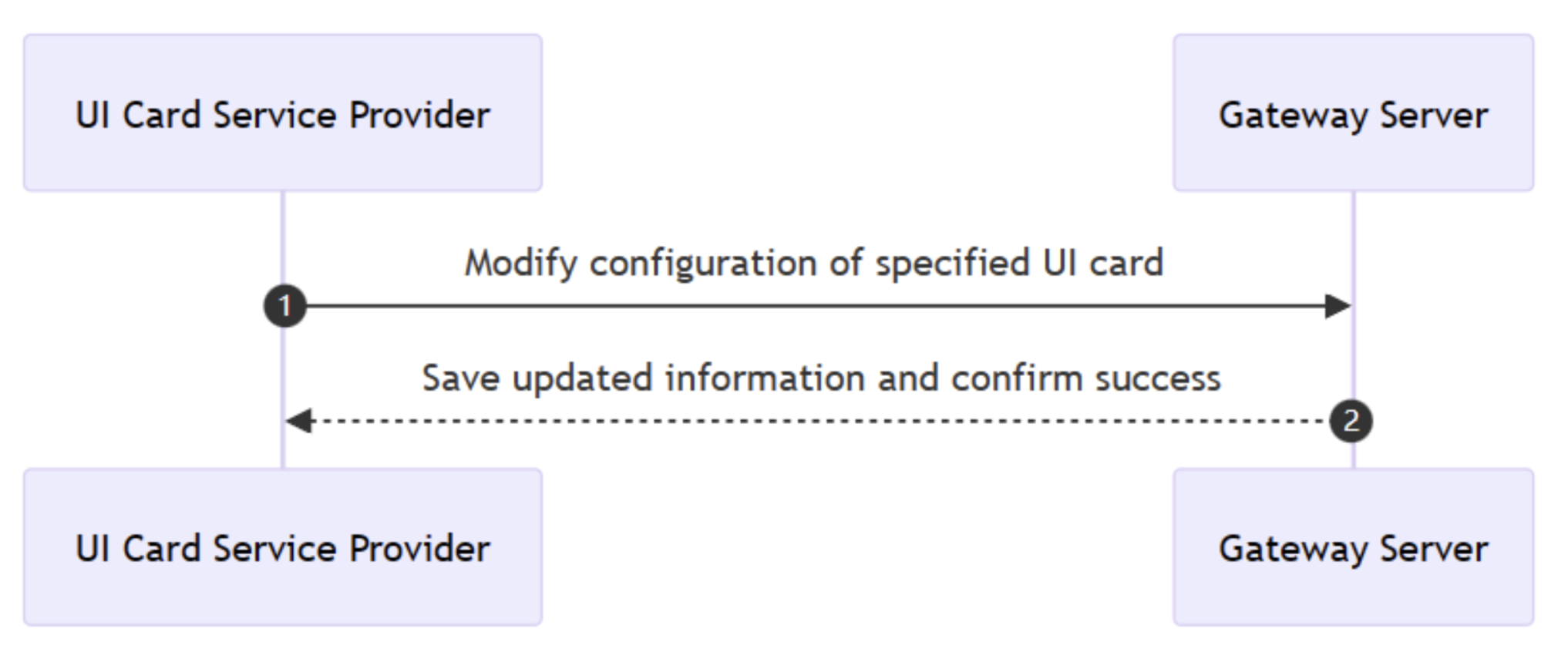
8.1.4 Deleting a Custom UI Card
- [UI Service Provider]: Calls the API to delete a specified custom UI card.
- [Gateway Server]: The gateway will remove all information related to the specified UI card.
8.2 Custom UI Card Module
8.2.1 Creating a Custom UI Card
The UI Service Provider sends a request to the gateway to create a custom UI card.
- URL:
/open-api/v2/rest/ui/cards
- Method:
POST
- Header:
-
- Content-Type: application/json
- Authorization: Bearer <token>
Request Parameters:
Attribute |
Type |
Optional |
Description |
label |
string |
Y |
Card Label: Used to describe the card. It is the card’s alias. |
cast_settings |
CastSettingsObject |
Y |
Cast Card Settings: Configuration settings for cast cards. |
web_settings |
WebSettingsObject |
N |
Web Card Settings: Configuration settings for web cards. |
CastSettingsObject:
Attribute |
Type |
Optional |
Description |
dimensions |
DimensionObject [] |
N |
Size Configuration: Must include at least one configuration. |
default |
string |
N |
Default Specification: The default size specification is used, with optional parameters: 2×2 |
WebSettingsObject:
Attribute |
Type |
Optional |
Description |
dimensions |
DimensionObject [] |
N |
Size Configuration: Must include at least one configuration. |
drawer_component |
DrawerObject |
Y |
Drawer Component Display Settings. |
default |
string |
N |
Default Specification:
|
DimensionObject:
Attribute |
Type |
Optional |
Description |
src |
string |
N |
Resource URL: For example: http://example.html |
size |
string |
N |
Size Specifications: CastSettingsObject Optional Parameters:
WebSettingsObject Optional Parameters:
|
DrawerObject:
Attribute |
Type |
Optional |
Description |
src |
string |
N |
Resource URL: For example: http://example.html |
Successful data response:
Attribute |
Type |
Optional |
Description |
id |
string |
N |
UI Card unique ID |
Conditions: The request parameters are legal, and the user identity verification is passed.
Status Code: 200 OK
Request Demo:
{
"label": "ewelink cube card",
"cast_settings": {
"dimensions": [
{
"src": "https://ewelink.cc/ewelink-cube/",
"size": "2×2"
}
],
"default": "2×2"
},
"web_settings": {
"dimensions": [
{
"src": "https://ewelink.cc/ewelink-cube/",
"size": "2×1"
},
{
"src": "https://ewelink.cc/ewelink-cube/",
"size": "1×1"
}
],
"drawer_component": {
"src": "https://ewelink.cc/ewelink-cube/"
},
"default": "2×1"
}
}
Response Example:
{
"error": 0,
"data": {
"id": "72cc5a4a-f486-4287-857f-b482d7818b16"
},
"message": "success"
}
8.2.2 Retrieve UI Card List
- URL:
/open-api/v2/rest/ui/cards
- Method:
GET
- Header:
-
- Content-Type: application/json
- Authorization: Bearer <token>
Request Parameters: None
Response Parameters:
Attribute |
Type |
Optional |
Description |
data |
CardObject[] |
N |
UI Card list |
CardObject:
Attribute |
Type |
Optional |
Description |
id |
string |
N |
Card ID: A unique identifier for the card. |
label |
string |
Y |
Card Label: Used to describe the card. It serves as an alias or name for the card. |
cast_settings |
CastSettingsObject |
Y |
Card Label: Used to describe the card. It is the card’s alias. |
web_settings |
WebSettingsObject |
N |
Cast Card Settings: Configuration settings for cast cards. |
app_name |
string |
Y |
Web Card Settings: Configuration settings for web cards. |
CastSettingsObject:
Attribute |
Type |
Optional |
Description |
dimensions |
DimensionObject [] |
N |
Size Configuration: Must include at least one configuration. |
default |
string |
N |
Default Specification: Optional Parameter: 2×2 |
used |
string |
N |
Current Specification: Optional Parameter: 2×2 |
WebSettingsObject:
Attribute |
Type |
Optional |
Description |
dimensions |
DimensionObject [] |
N |
Size Configuration: Must include at least one configuration. |
drawer_component |
DrawerObject |
Y |
Drawer Component Display Settings. |
default |
string |
N |
Default Specification: Optional Parameter:
|
used |
string |
N |
Current Specification: Optional Parameter:
|
DimensionObject:
Attribute |
Type |
Optional |
Description |
src |
string |
N |
Resource URL: For example: http://example.html |
size |
string |
N |
Size Specifications: Optional Parameter:
Note: Currently, cast cards only support the 2×2 specification. The 2×2 specification will not be effective. |
DrawerObject:
Attribute |
Type |
Optional |
Description |
src |
string |
N |
Resource URL: For example: http://example.html |
Response Example:
{
"error": 0,
"data": [
{
"id": "72cc5a4a-f486-4287-857f-b482d7818b16",
"label": "ewelink cube card",
"cast_settings": {
"dimensions": [
{
"src": "https://ewelink.cc/ewelink-cube/",
"size": "2×2"
}
],
"default": "2×2",
"used": "2×2"
},
"web_settings": {
"dimensions": [
{
"src": "https://ewelink.cc/ewelink-cube/",
"size": "2×1"
},
{
"src": "https://ewelink.cc/ewelink-cube/",
"size": "1×1"
}
],
"drawer_component": {
"src": "https://ewelink.cc/ewelink-cube/"
},
"default": "2×1",
"used": "2×1"
},
"appName": "ewelink-cube"
}
],
"message": "success"
}
8.2.3 Modify Configuration of a Specified UI Card
Authorized users are allowed to modify the configuration of an existing UI card through this interface. Custom card service providers can only modify UI cards they have created.
- URL:
/open-api/v2/rest/ui/cards/{id}
- Method:
PUT
- Header:
-
- Content-Type: application/json
- Authorization: Bearer <token>
Request Parameters:
Attribute |
Type |
Optional |
Description |
label |
string |
Y |
Used to describe the card. It is the card’s alias. |
cast_settings |
CastSettingsObject |
Y |
Cast Card Settings |
web_settings |
WebSettingsObject |
Y |
Web Card Settings |
CastSettingsObject:
Attribute |
Type |
Optional |
Description |
used |
string |
Y, Either |
Current Specification: Optional Parameter:
|
src |
string |
Y, Either |
Resource URL: http://example.html |
WebSettingsObject:
Attribute |
Type |
Optional |
Description |
used |
string |
Y, Either |
Current Specification: Optional Parameter:
|
src |
string |
Y, Either |
Resource URL: http://example.html |
Successful data response:
Conditions: The request parameters are legal, and the user identity verification is passed. The UI card being modified must be created by the custom UI card service provider calling the interface.
Status Code: 200 OK
Error Code:
- 406: No permission to access this resource
Response Example:
{
"error": 0,
"data": {},
"message": "success"
}
Request Example:
{
"cast_settings": {
"used": "2×2"
},
"web_settings": {
"used": "1×1"
}
}
8.2.4 Delete Custom UI Card
Authorized users are permitted to delete an existing UI card using this interface. Custom card service providers can only delete UI cards that they have created.
- URL:
/open-api/v2/rest/ui/cards/{id}
- Method:
DELETE
- Header:
-
- Content-Type: application/json
- Authorization: Bearer <token>
Request Parameters: None
Successful data response:
Conditions: The request parameters are legal, and the user identity verification is passed. The UI card being modified must be created by the custom UI card service provider calling the interface.
Status Code: 200 OK
Error Code:
- 406: No permission to access this resource.
Response Example:
{
"error": 0,
"data": {},
"message": "success"
}